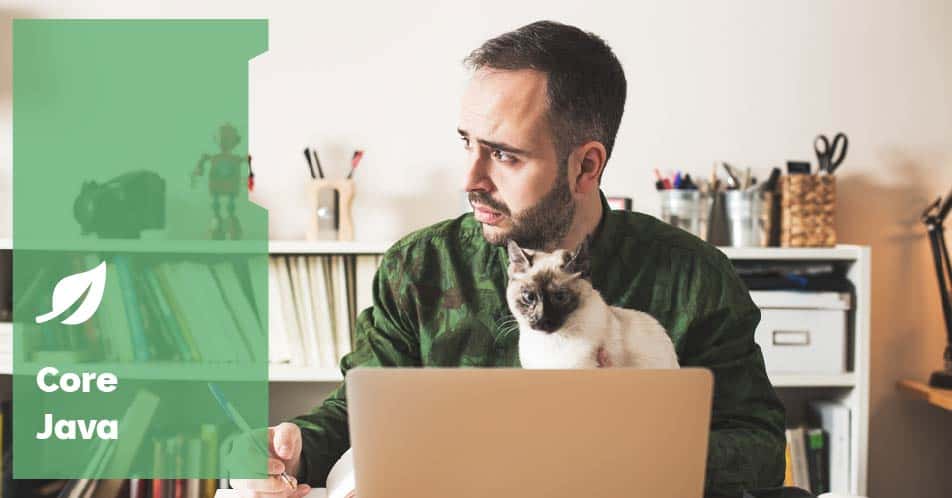
1. Overview
In many applications, we may need to display only the last two digits of the current year rather than the full four-digit year. In this tutorial, we’ll examine three distinct methods to do this:
- using the legacy Calendar class with a modulo operation
- leveraging SimpleDateFormat for automatic formatting
- harnessing the modern java.time API
2. Using Calendar and the Modulo Operator
Before we begin testing our approaches, we define a constant that holds the expected two-digit year format:
String EXPECTED = Year.now().format(DateTimeFormatter.ofPattern("uu"));
This constant guarantees that every method uses the same reference value for comparison.
This approach uses the legacy Calendar API to retrieve the current year and applies the modulo operator to extract the last two digits:
@Test
public void whenUsingCalendarModulo_thenReturnsCorrectTwoDigitYear() {
int currentYear = Calendar.getInstance().get(Calendar.YEAR);
int lastTwo = currentYear % 100;
String result = (lastTwo < 10) ? "0" + lastTwo : String.valueOf(lastTwo);
assertEquals(EXPECTED, result);
}
We need to note that this solution has the disadvantage of requiring us to add a leading 0 when the extracted number is less than 10.
3. Using SimpleDateFormat
The SimpleDateFormat class allows to format a Date based on a given pattern. Here, the “yy” pattern automatically formats the year to two digits (adding a leading zero when necessary):
@Test
public void whenUsingSimpleDateFormat_thenReturnsCorrectTwoDigitYear() {
DateFormat df = new SimpleDateFormat("yy");
String result = df.format(new Date());
assertEquals(EXPECTED, result);
}
The SimpleDateFormat approach streamlines our code by automatically adding a leading zero, so we avoid extra conditional logic.
4. Using java.time API
Using the modern java.time API, we can simplify date and time handling by directly formatting the current year. We utilize the Year class along with a DateTimeFormatter configured with the “uu” pattern, which provides a two-digit year in a concise and thread-safe manner:
@Test
public void whenUsingJavaTimeAPI_thenReturnsCorrectTwoDigitYear() {
String result = Year.now().format(DateTimeFormatter.ofPattern("uu"));
assertEquals(EXPECTED, result);
}
This approach improves readability, and is inherently thread-safe, making it ideal for modern Java applications.
5. Conclusion
In this article, we examined three methods for displaying the last two digits of the current year in Java.
We started by using the Calendar API with the modulo operator. This method works well but requires us to add a leading zero manually for single-digit results.
Next, we used SimpleDateFormat, which streamlines our code by automatically formatting the year with a leading zero.
Finally, we leveraged the modern java.time API. This approach improves readability, ensures thread safety, and simplifies date handling.
As always, the source code is available over on GitHub.
The post How to Display the Last Two Digits of the Current Year in Java first appeared on Baeldung.