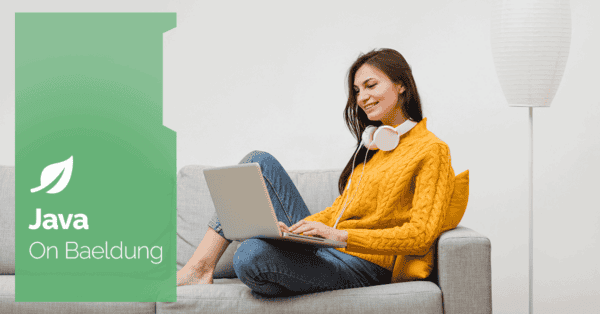
1. Introduction
In Java, a LinkedList is a commonly used data structure that allows dynamic memory allocation and efficient insertions or deletions. There are multiple ways to print a LinkedList’s elements, such as loops, iterators, or streams. One convenient approach is using the toString() method.
The toString() method provides a simple and readable way to display the contents of a LinkedList without manually iterating through its elements.
In this tutorial, we’ll understand how to print a LinkedList using Java’s toString() method.
2. What is the LinkedList.toString()
The toString() method is inherited from the AbstractCollection class and is overridden in the LinkedList class. This method automatically formats the output in a structured manner, making debugging and logging easier.
Additionally, it reduces code complexity and improves maintainability by eliminating the need for explicit iteration logic. The toString() method doesn’t accept any argument:
public String toString()
It returns a human-readable string representation of the list.
2.1. Key Advantages of Printing LinkedList Using toString()
The toString() method offers several advantages, which simplifies LinkedList printing without manual iteration:
- It ensures code clarity and eliminates the need for complex loops or iteration logic
- The formatted string output is useful for debugging, as it clearly displays the elements of the LinkedList, which helps in quick issue identification
- The default toString() returns output in a consistent format that is universally understandable. This makes it ideal for logging or displaying list contents in a standard manner
However, the default toString() implementation may not format the output as needed. In such cases, we can customize the method to enhance the representation of LinkedList elements.
3. How to Print a LinkedList Using toString() Method
We can invoke the toString() method on a LinkedList to print its content in string representation.
In this example, we first create a non-empty LinkedList called idsList and add some integer values to it. Also, we created an empty list named emptyList:
LinkedList<Integer> idsList = new LinkedList<>();
idsList.add(101);
idsList.add(102);
idsList.add(103);
LinkedList<Integer> emptyList = new LinkedList<>();
Next, we use the toString() method to print a LinkedList named idsList explicitly:
assertEquals("[101, 102, 103]", idsList.toString(),
"Default LinkedList toString() should match expected format.");
We also invoke the toString() method on an empty list named emptyList to print it:
assertEquals("[]", emptyList.toString(),
"Empty LinkedList should return empty brackets.");
The toString() method returns a string representation of the idsList, with elements enclosed in square brackets and separated by commas. Moreover, it returns [] for emptyList. Notably, printing idsList with println(idsList) returns the same result as println(idsList.toString()) because the println() method automatically calls toString() on the list.
4. How to Customize the toString() Method
Sometimes, the default toString() output may not fit our formatting needs. In those scenarios, we can easily customize the toString() method to customize the output format.
Moreover, customizing the toString() method gives more control over how the list’s elements are displayed, making it useful for debugging complex data structures.
Let’s customize the toString() function to display elements with a customized separator “-“. We create a customToString() method that enables us to customize how a LinkedList of integers is displayed. We remove the last ” – ” using the delete() method:
public static String customToString(LinkedList<Integer> list) {
StringBuilder sb = new StringBuilder();
sb.append("Custom LinkedList: ");
if (list.isEmpty()) {
sb.append("Empty List");
} else {
for (Integer item : list) {
sb.append(item).append(" - ");
}
sb.delete(sb.length() - 3, sb.length());
}
return sb.toString();
}
Let’s test the customToString() method to ensure it formats the list elements correctly using a ” – ” separator:
String expected = "Custom LinkedList: 101 - 102 - 103";
assertEquals(expected, NewLinkedList.customToString(idsList),
"Custom toString() should format the list elements with ' - ' separator.");
The test returns “Empty List” when the list is empty; otherwise, it iterates through the list and formats the elements with ” – ” between them:
Custom LinkedList: 101 - 102 - 103
Custom LinkedList: Empty List
5. Best Practices for Using the toString() Method
If the default output format (i.e., elements enclosed in [] and separated by commas) is sufficient, we should avoid customizing the method unnecessarily. However, if we need a specific format, we should customize toString() or create a helper method.
When a LinkedList stores complex objects, calling toString() on each object can produce excessive or unclear output, in such a case, it’s recommended to customize toString() in the object’s custom class. To optimize performance, we should use StringBuilder instead of string concatenation (+) when customizing toString(). Additionally, the custom toString() output should be clear and consistent, especially for debugging or logging.
6. Conclusion
In this article, we explored how to print a LinkedList using Java’s toString() method. This method provides a convenient display of list elements, making debugging and logging easier.
We also discussed its advantages and limitations and how to customize it for custom formatting. Additionally, we covered best practices to ensure efficient and readable output. By following these practices, we can effectively use the toString() method to print out a LinkedList.
Finally, the source code for all examples is available over on GitHub.
The post Printing out a LinkedList Using toString() first appeared on Baeldung.