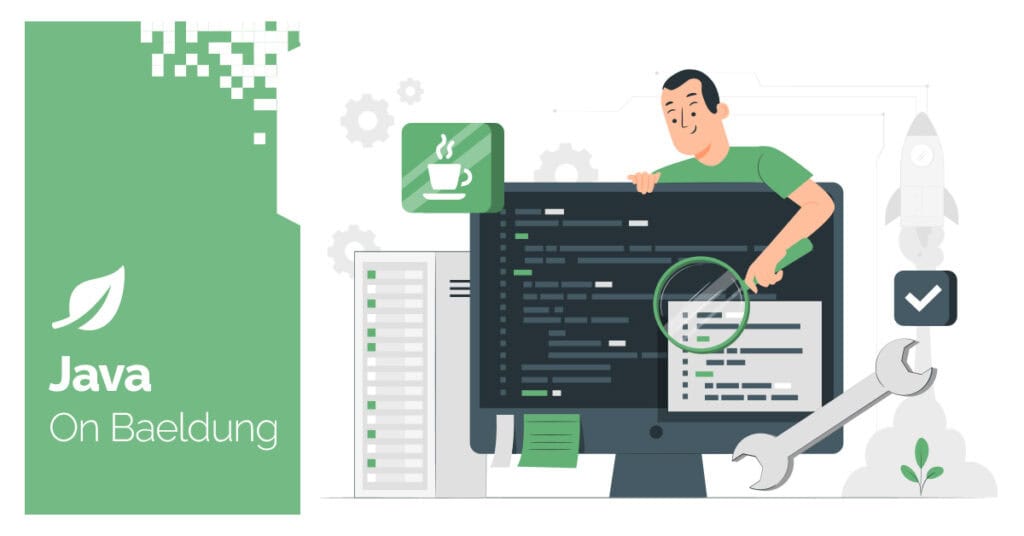
1. Introduction
In this article, we’ll explore various methods for checking if all values in a Java Map are the same. We’ll examine methods such as conventional loops and the Stream API while evaluating their effectiveness and readability.
The practical examples will give us insights into choosing the most effective solution for our applications.
2. Checking if All Values in a Map Are the Same
We’ll review four main strategies. Each uses a different technique to verify if all values in a map are the same. Each strategy has benefits and limitations, so let’s discuss each one in detail.
Let’s create a simple map object that we’ll use throughout the strategies:
Map<String, Boolean> map = Map.of("English", 100, "Maths", 100, "Science", 100);
2.1. Using allMatch() With Streams
The allMatch() method, part of Java 8’s Stream API, checks if all elements in a collection satisfy a specified condition. In our case, it checks if all values in the map are equal to the first value in the collection:
boolean areAllValuesSameWithAllMatch(Map<String, Integer> map) {
int firstValue = map.values().iterator().next();
return map.values().stream().allMatch(value -> value.equals(firstValue));
}
Here, we get the first value from the map and use allMatch() to verify if every value matches this firstValue. If all values are the same, it returns true. Otherwise, it stops as soon as a different value is found.
2.2. Using a Set to Check for Unique Values
By collecting all values into a HashSet, we take advantage of the fact that the Set automatically removes duplicates:
boolean areAllValuesSameWithSet(Map<String, Integer> map) {
Set<Integer> uniqueValues = new HashSet<>(map.values());
return uniqueValues.size() == 1;
}
Here, we collect all values in the Map into a HashSet, which automatically filters out duplicate values. Finally, we check the size of the HashSet. If it’s 1, all values are the same. If it’s greater than 1, there are different values in the Map.
2.3. Using Stream.reduce() With Boolean.logicalAnd
In this method, we use Java Streams reduce() function to combine the values using a logical AND operation, comparing each one to the first value. This method works well if we prefer the functional programming style and don’t require early termination:
boolean areAllValuesSameWithReduce(Map<String, Integer> map) {
int firstValue = map.values().iterator().next();
return map.values().stream().reduce(true,
(result, value) -> result && value.equals(firstValue),
Boolean::logicalAnd);
}