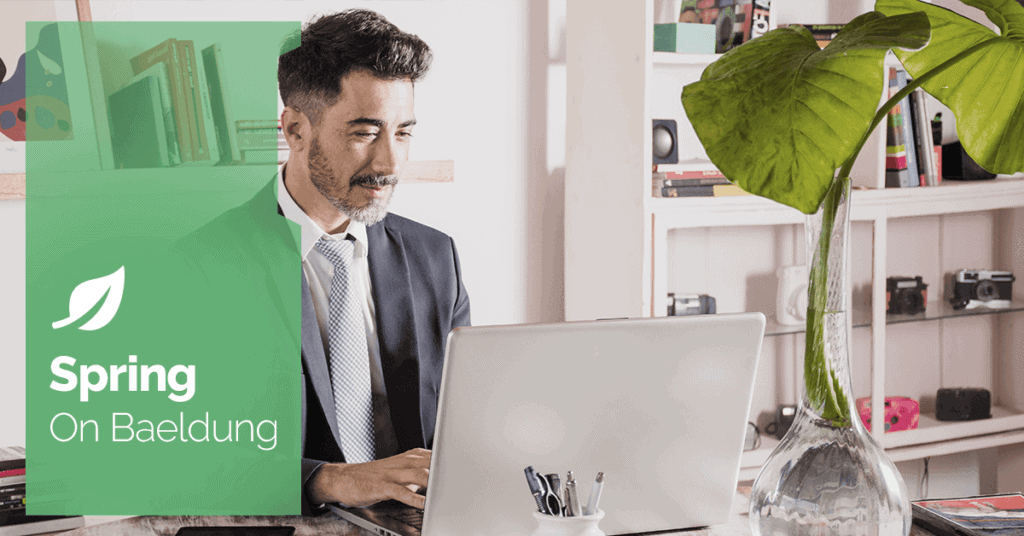
1. Overview
Most businesses now rely on websites and web applications, with nearly every organization operating online. Since they connect with end users and customers, websites and web apps must work flawlessly across all popular browsers, browser versions, and operating systems.
Though manual testing can get the job done, automated testing is the better choice for speed and efficiency in testing. Automation testing tools like Selenium allow businesses to run automated browser tests, enabling them to deliver high-quality websites and web apps more quickly.
In this article, we’ll learn how to perform automated browser testing with Selenium.
2. What Is Selenium?
Selenium is an open-source tool that helps automate web browser tests. It provides benefits such as cross-browser testing, support for multiple programming languages, platform independence, huge community, etc.
The recent introduction of Selenium Manager provides automated browser and driver management that allows developers and testers to seamlessly run the Selenium tests without worrying about downloading and installing the drivers and browsers.
3. Why Selenium for Automated Browser Testing?
The following are the major reasons for using Selenium for automated browser testing:
- Selenium is a free and open-source tool that offers a cost-effective solution for web automation testing.
- It supports multiple popular programming languages such as Java, Python, JavaScript, C#, Ruby, and PHP.
- Selenium enables software teams to test their websites and web applications on various browsers such as Chrome, Firefox, Edge, and Safari.
- It has a large, active community that offers extensive support and resources.
4. How to Perform Browser Automation With Selenium?
There are a few prerequisites, setup, and execution processes that we need to follow depending on the programming language selected for writing test automation scripts using Selenium.
4.1. Prerequisites
Here are some prerequisites to get started with automated browser testing with Selenium:
- Download and install Java JDK
- Install IntelliJ or Eclipse IDE
- Download the required Maven build tools
We’ll not download the browsers since the tests will be run on cloud-based testing platforms such as LambdaTest. It’s an AI-powered test execution platform that enables developers and testers to perform automation testing with Selenium at scale across 3000+ real browsers, browser versions, and operating systems.
4.2. Setup and Configuration
Let’s add the following Selenium WebDriver dependency and TestNG dependency in the pom.xml file:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.25.0</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.10.2</version>
<scope>test</scope>
</dependency>
As we’ll run the tests on the LambdaTest platform, we need to add certain capabilities for the platform, browser, and browser versions. For this, let’s use the LambdaTest Automation Capabilities Generator.
Let’s create a BaseTest.java class to add all the configuration details in it:
public class BaseTest {
private static final ThreadLocal<RemoteWebDriver> DRIVER =
new ThreadLocal<>();
//...
}
ThreadLocal instance in the BaseTest class is for thread safety, as we’ll run the tests in parallel. It also ensures that each thread has its instance of RemoteWebDriver, facilitating smooth parallel test execution.
Next, the getDriver() and setDriver() methods will get and set the instance of RemoteWebDriver using the ThreadLocal instance. We’ll set the LambdaTest automation capabilities using the getLtOptions() method:
public RemoteWebDriver getDriver() {
return DRIVER.get();
}
private void setDriver(RemoteWebDriver remoteWebDriver) {
DRIVER.set(remoteWebDriver);
}
private HashMap<String, Object> getLtOptions() {
HashMap<String, Object> ltOptions = new HashMap<>();
ltOptions.put("project", "ECommerce playground website");
ltOptions.put("build", "LambdaTest Ecommerce Website tests");
ltOptions.put("name", "Automated Browser Testing");
ltOptions.put("w3c", true);
ltOptions.put("visual", true);
ltOptions.put("plugin", "java-testNG");
return ltOptions;
}
Using the getLtOptions() method, we’ll set the project, build and test names, and enable the java-testNG plugin in the LambdaTest cloud environment. Next, we’ll add the getChromeOptions() method that will hold the capabilities for the Chrome browser and will return the ChromeOptions.
Similarly, the getFirefoxOptions() method accepts the browserVersion and platform as parameters, sets its respective capabilities, and returns the FirefoxOptions.
In the BaseTest class, we’ll use the setup() method to set up Selenium WebDriver to run automated tests on both Chrome and Firefox. The @BeforeTest annotation ensures the method runs before any tests, while @Parameters passes browser, browser version, and platform values from the testng.xml file at runtime. This helps run cross-browser tests in parallel on Chrome and Firefox:
if (browser.equalsIgnoreCase("chrome")) {
try {
setDriver(new RemoteWebDriver(new URL("http://" + userName + ":" + accessKey + gridUrl),
getChromeOptions(browserVersion, platform)));
} catch (final MalformedURLException e) {
throw new Error("Could not start the Chrome browser on the LambdaTest cloud grid");
}
} else if (browser.equalsIgnoreCase("firefox")) {
try {
setDriver(new RemoteWebDriver(new URL("http://" + userName + ":" + accessKey + gridUrl),
getFirefoxOptions(browserVersion, platform)));
} catch (final MalformedURLException e) {
throw new Error("Could not start the Firefox browser on the LambdaTest cloud grid");
}
}
To connect to the LambdaTest cloud grid, we need to have the LambdaTest Username, Access Key, and grid URL. We can easily find the LambdaTest Username and Access Key from our Account Settings > Password & Security.
After that, we’ll write the code for handling the browsers and starting their respective sessions.
4.3. Test Scenario
Let’s use the following test scenario to demonstrate the automated browser testing with Selenium on the LambdaTest platform.
- Navigate to the LambdaTest eCommerce Playground website.
- Locate and click on the Shop by Category menu on the home page.
- Locate and click on the MP3 Players menu.
- Assert that the page displayed shows the page header MP3 Players.
4.4. Test Implementation
Let’s create an AutomatedBrowserTest.java class to write the test automation scripts for the test scenario. This class extends the BaseTest class for code reuse and modularity.
Then, we’ll create the whenUserNavigatesToMp3PlayersPage_thenPageHeaderShouldBeCorrectlyDisplayed() method in the AutomatedBrowserTest class that implements the test scenario:
@Test
public void whenUserNavigatesToMp3PlayersPage_thenPageHeaderShouldBeCorrectlyDisplayed() {
getDriver().get("https://ecommerce-playground.lambdatest.io/");
HomePage homePage = new HomePage(getDriver());
homePage.openShopByCategoryMenu();
Mp3PlayersPage mp3PlayersPage = homePage.navigateToMp3PlayersPage();
assertEquals(mp3PlayersPage.pageHeader(), "MP3 Players");
}
We have used the Selenium WebDriver Page Object pattern, which helps in better code readability and maintainability. The HomePage class holds all the page objects of the home page of the LambdaTest eCommerce Playground website.
The openShopByCategoryMenu() method locates the Shop By Category menu using the linkText locator strategy of Selenium and performs a click on it. This MP3 Players menu is located using the CSS Selector #widget-navbar-217841 > ul > li:nth-child(5) > a. Then, the navigateToMP3PlayersPage() method locates the MP3 Players page, performs a click on it, and returns a new instance of the MP3PlayersPage class.
The MP3PlayersPage class holds all the objects of the MP3 Player page:
public class Mp3PlayersPage {
private WebDriver driver;
public Mp3PlayersPage(WebDriver driver) {
this.driver = driver;
}
public String pageHeader() {
return driver.findElement(By.cssSelector(".content-title h1"))
.getText();
}
}
4.5. Test Execution
Let’s create a new testng.xml file to run tests in parallel. This file configures TestNG to run automated browser tests on Chrome and Firefox on Windows 10.
Now, let’s run the tests and visit the LambdaTest Web Automation dashboard to check test execution results.
Here’s a snap of test execution on the Chrome browser:
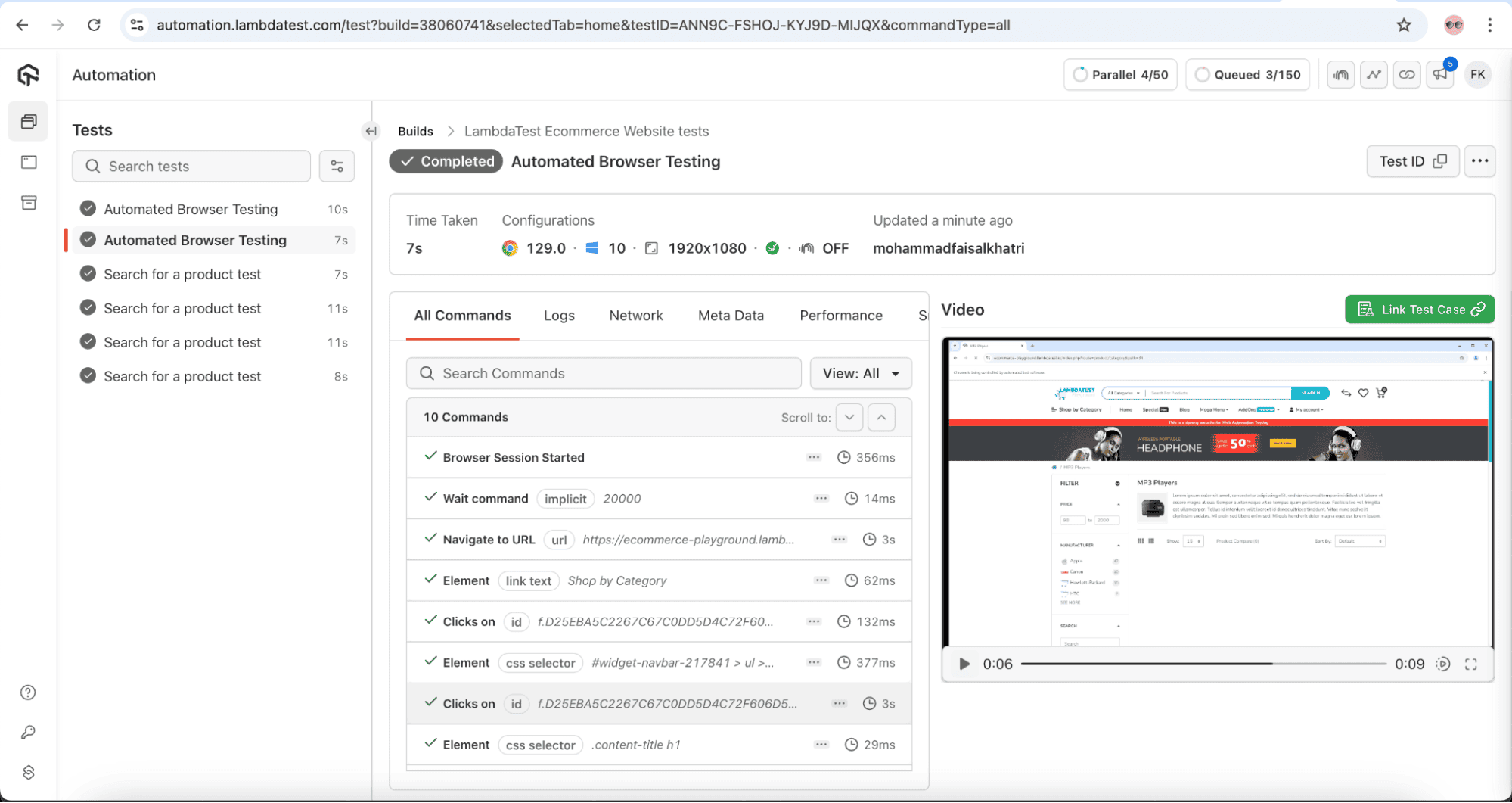
And here’s a snap of test execution on the Firefox browser:
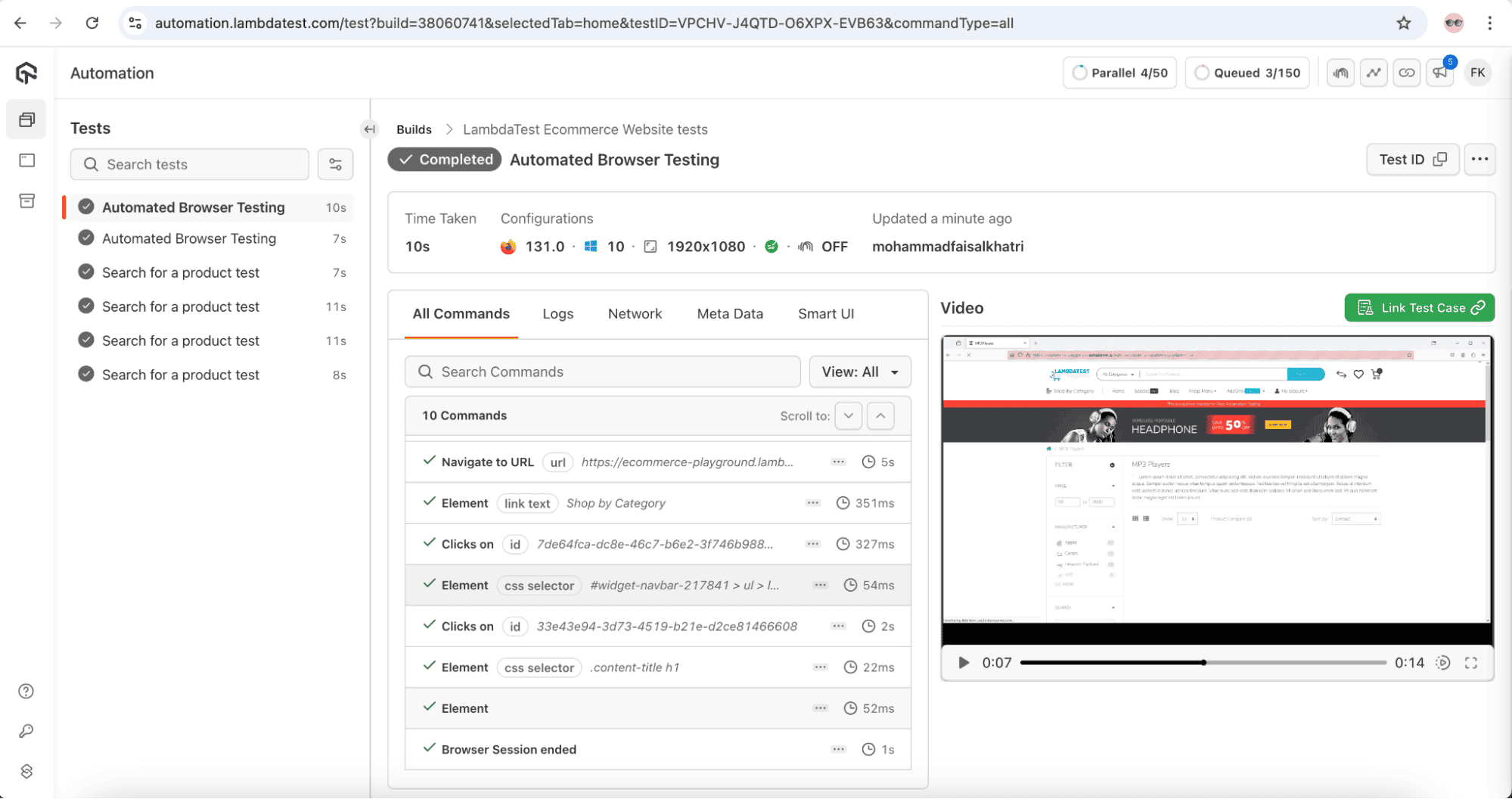
We can find all granular details of the tests, including the browser name, version, platform, and logs of test execution, as well as video recordings and screenshots.
5. Conclusion
To sum up, automated browser testing plays a crucial role in quickly testing websites and web applications. With Selenium, testers can efficiently run multiple tests at the same time, leading to faster feedback. This method not only helps identify issues early but also ensures that the software remains stable.
The source code used in this tutorial is available over on GitHub.