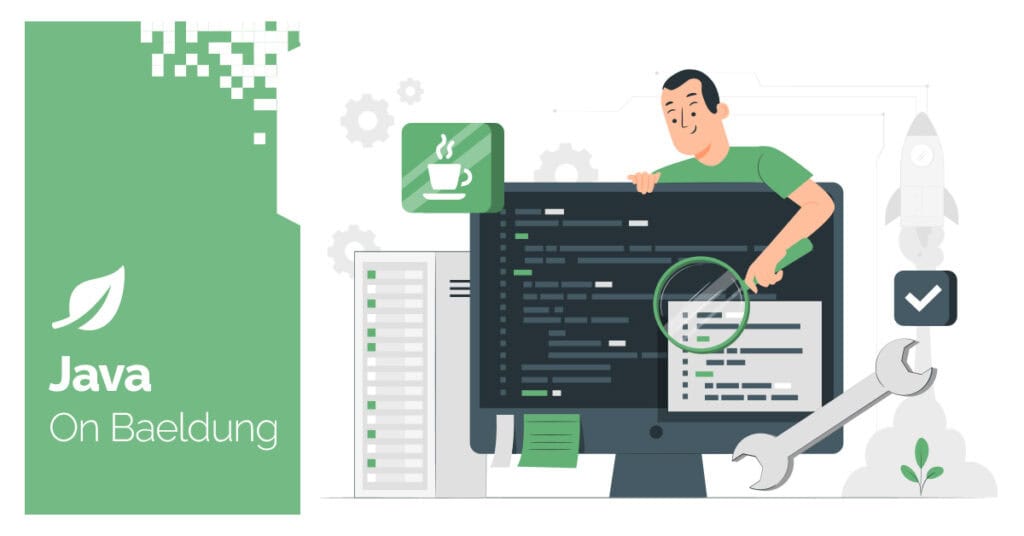
1. Introduction
In this tutorial, we’ll explore how to filter a list that contains nested lists in Java. When working with complex data structures, such as lists of objects that contain other lists, it becomes essential to extract specific information based on certain criteria.
2. Understanding the Problem
We’ll work with a simple example where we have a User class and an Order class. The User class has a name and a list of Orders, while the Order class has a product and a price. Our goal is to filter a list of users based on some conditions applied to their nested orders.
Below is how our data model is structured:
class User {
private String name;
private List<Order> orders;
public User(String name, List<Order> orders) {
this.name = name;
this.orders = orders;
}
// set get methods
}
class Order {
private String product;
private double price;
public Order(String product, double price) {
this.product = product;
this.price = price;
}
// set get methods
}
In this setup, each User can have multiple Order objects, which provide the details necessary for us to filter the users based on certain criteria related to their orders, such as price.
To demonstrate the filtering logic, we’ll first create some sample test data. In the example below, we prepare two Order objects and associate them with three User objects:
Order order1 = new Order("Laptop", 600.0);
Order order2 = new Order("Phone", 300.0);
Order order3 = new Order("Monitor", 510.0);
Order order4 = new Order("Monitor", 200.0);
User user1 = new User("Alice", Arrays.asList(order1, order4));
User user2 = new User("Bob", Arrays.asList(order3));
User user3 = new User("Mity", Arrays.asList(order2));
List users = Arrays.asList(user1, user2, user3);
Suppose we want to find all users who have placed orders with a price greater than $500. In this case, we’d expect our filtering logic to return two users, as Alice and Bob both have an order meeting this criterion.
3. Traditional Looping Approach
Before Java 8 introduced the Stream API, the typical way to filter lists was by using traditional for loops. Let’s take the same example and implement filtering using nested for loops:
double priceThreshold = 500.0;
List<User> filteredUsers = new ArrayList<>();
for (User user : users) {
for (Order order : user.getOrders()) {
if (order.getPrice() > priceThreshold) {
filteredUsers.add(user);
break;
}
}
}
assertEquals(2, filteredUsers.size());
In this approach, we loop through each User and then loop through their list of Orders. As soon as we find an order that matches our condition, we add the user to the filtered list and exit the inner loop with a break.
This approach works fine and is easy to understand, but it requires manual management of the nested loops. It also lacks the functional programming style that streams provide.
4. Filtering Using Java Streams
With Java 8, we can use Streams for cleaner code. Let’s use this approach to solve the same problem as before: We want to filter out the users who have placed an order with a price of more than $500. We can do this by using Java Streams to check each user’s list of orders to see if it contains an order meeting our condition:
double priceThreshold = 500.0;
List<User> filteredUsers = users.stream()
.filter(user -> user.getOrders().stream()
.anyMatch(order -> order.getPrice() > priceThreshold))
.collect(Collectors.toList());
assertEquals(2, filteredUsers.size());
In this code, we’re streaming through the list of Users and then, for each user, we’re checking if any of their orders have a product price of more than $500. The anyMatch() method helps us check if at least one of the user’s orders had paid more than $500.
5. Filtering With Multiple Conditions
Now, suppose we want to filter users who ordered a “Laptop” and also paid more than $500. In this case, we can combine conditions inside the anyMatch() method:
double priceThreshold = 500.0;
String productToFilter = "Laptop";
List<User> filteredUsers = users.stream()
.filter(user -> user.getOrders().stream()
.anyMatch(order -> order.getProduct().equals(productToFilter)
&& order.getPrice() > priceThreshold))
.collect(Collectors.toList());
assertEquals(1, filteredUsers.size());
assertEquals("Alice", filteredUsers.get(0).getName());
Here, we expect that only user1 will be included in the filteredUsers list, as Alice is the only user who has ordered a “Laptop” and paid more than $500.
6. Using a Custom Predicate
Another approach is to encapsulate our filtering logic in a custom Predicate. This allows for more readable and reusable code. Let’s define a Predicate that checks whether a user’s order matches our condition:
Predicate<User> hasExpensiveOrder = user -> user.getOrders().stream()
.anyMatch(order -> order.getPrice() > priceThreshold);
List<User> filteredUsers = users.stream()
.filter(hasExpensiveOrder)
.collect(Collectors.toList());
assertEquals(2, filteredUsers.size());
This method improves readability by keeping the filtering logic isolated, and the predicate can be reused for other filtering operations if needed.
7. Filtering While Preserving Structure
Let’s consider the scenario where we want to keep all users on the list but only include orders that meet a certain condition. Instead of removing users without valid orders, we’re modifying their list of orders and filtering out only the orders that don’t meet the condition.
In this case, we need to modify the user’s list of orders while preserving the rest of the user object:
List<User> filteredUsersWithLimitedOrders = users.stream()
.map(user -> {
List<Order> filteredOrders = user.getOrders().stream()
.filter(order -> order.getPrice() > priceThreshold)
.collect(Collectors.toList());
user.setOrders(filteredOrders);
return user;
})
.filter(user -> !user.getOrders().isEmpty())
.collect(Collectors.toList());
assertEquals(2, filteredUsersWithLimitedOrders.size());
assertEquals(1, filteredUsersWithLimitedOrders.get(0).getOrders().size());
assertEquals(1, filteredUsersWithLimitedOrders.get(1).getOrders().size());
Here, we’re using map() to modify each User object. For every user, we filter their list of orders to include only those that meet the price condition, and then, we update their order list with the filtered results.
8. Using flatMap()
Instead of using nested streams, we can leverage the flatMap() method to flatten the nested lists and process the items as a single stream. This approach simplifies filtering across nested lists by avoiding multiple stream() calls.
Let’s see how we can use flatMap() to filter User objects based on their Order list:
List<User> filteredUsers = users.stream()
.flatMap(user -> user.getOrders().stream()
.filter(order -> order.getPrice() > priceThreshold)
.map(order -> user))
.distinct()
.collect(Collectors.toList());
assertEquals(2, filteredUsers.size());
In this approach, we use the flatMap() method to transform each User into a stream of their associated Order objects. By doing so, we can process all orders as a single unified stream.
9. Handling Edge Cases
There may be cases where some users have no orders at all. To prevent potential NullPointerExceptions when handling users without any orders, we should implement checks to ensure that the orders are not null or empty:
User user1 = new User("Alice", Arrays.asList(order1, order2));
User user2 = new User("Bob", Arrays.asList(order3))
User user3 = new User("Charlie", new ArrayList<>());
List users = Arrays.asList(user1, user2, user3);
List<User> filteredUsers = users.stream()
.filter(user -> user.getOrders() != null && !user.getOrders().isEmpty())
.filter(user -> user.getOrders().stream()
.anyMatch(order -> order.getPrice() > priceThreshold))
.collect(Collectors.toList());
assertEquals(2, filteredUsers.size());
In this example, we’re first checking if the orders list is not null and not empty before applying further filters. This makes our code safer and avoids runtime errors.
10. Conclusion
In this article, we learned how to filter a list based on their nested lists in Java. We explored traditional loops, Java Streams, custom predicates, and how to handle edge cases. By using Streams, we can write cleaner and more efficient code.
As always, the code discussed here is available over on GitHub.