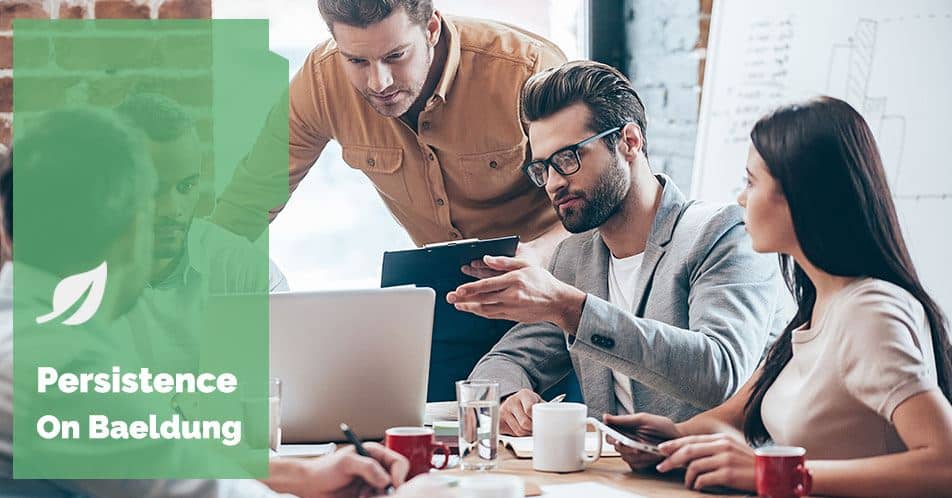
1. Overview
In Spring Data, it’s common to query entities using derived queries based on method names. When dealing with relationships between entities, such as nested objects, Spring Data provides various mechanisms to retrieve data from these nested objects.
In this tutorial, we’ll explore how to query by properties of nested objects using query derivation and JPQL (Java Persistence Query Language).
2. Scenario Overview
Let’s consider a simple scenario with two entities: Customer and Order. Each Order is linked to a Customer through a ManyToOne relationship.
We want to find all orders that belong to a customer with a specific email. In this case, the email is a property of the Customer entity, while our main query will be performed on the Order entity.
Here are our sample entities:
@Entity
@Table(name = "customers")
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// getters and setters
}
@Entity
@Table(name = "orders")
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Date orderDate;
@ManyToOne
@JoinColumn(name = "customer_id")
private Customer customer;
// getters and setters
}
3. Use Query Derivation
Spring Data JPA simplifies query creation by allowing developers to derive queries from method signatures in repository interfaces:
3.1. Normal Use Case
In normal cases, if we want to find Order entities by the email of the associated Customer, we can do this simply as:
public interface OrderRepository extends JpaRepository<Order, Long> {
List<Order> findByCustomerEmail(String email);
}
The generated SQL will look like:
select
o.id,
o.customer_id,
o.order_date
from
orders o
left outer join
customers c
on o.customer_id = c.id
where
c.email = ?
3.2. Conflicting Keyword Case
Now, suppose that, in addition to the nested Customer object, we also have a field called customerEmail in the Order class itself. In this case, Spring Data JPA wouldn’t generate a query on only the customers table as we would expect:
select
o.id,
o.customer_id,
o.customer_email,
o.order_date
from
orders o
where
o.customer_email = ?
In this case, we can use the underscore character to define where JPA should try to split the keyword:
List<Order> findByCustomer_Email(String email);
Here, the underscore helps Spring Data JPA parse the query method correctly.
4. Using a JPQL Query
If we want more control over our query logic or to perform more complex operations, JPQL is a good choice. To query Order by Customer‘s email using JPQL, we would write something like:
@Query("SELECT o FROM Order o WHERE o.customer.email = ?1")
List<Order> findByCustomerEmailAndJPQL(String email);
This gives us the flexibility to write more tailored queries without relying on method name conventions.
5. Conclusion
In this article, we’ve explored how to query data by properties of nested objects in Spring Data. We covered both query derivation and custom JPQL queries. By leveraging these techniques, we can handle many use cases with ease.
The example code from this tutorial can be found over on GitHub.