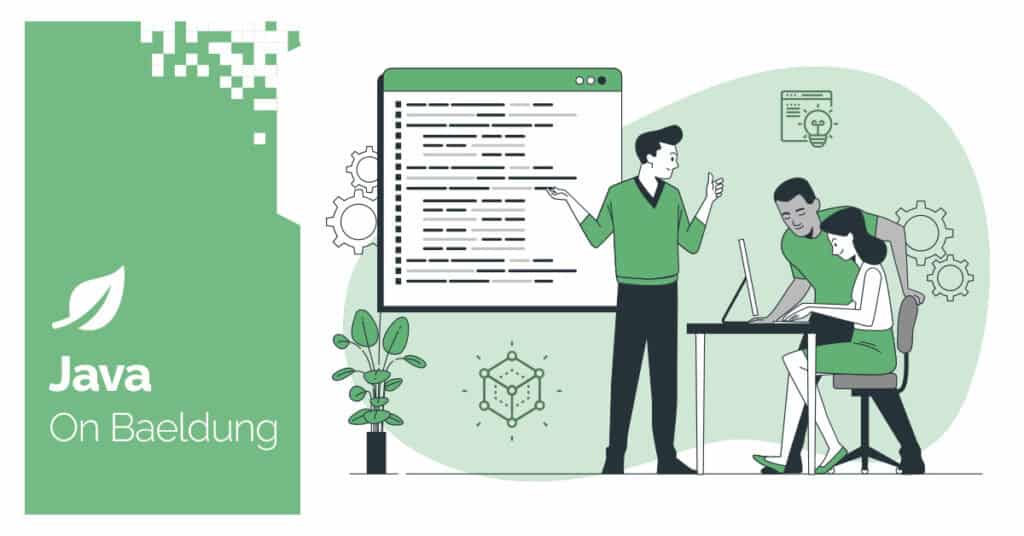
1. Introduction
In this tutorial, we’ll examine what distinguishes @EnableEurekaClient from @EnableDiscoveryClient.
Both are annotations used when working with the service registry in Spring Boot while developing microservices. These annotations become the base for other clients or microservices to register in the service registry as part of client-side discovery.
2. Service Registries in Microservices
Within the realm of microservices, characterized by their distributed and loosely coupled nature, we encounter a significant challenge. Specifically, as the number of services expands, maintaining an accurate inventory and ensuring seamless communication between them becomes increasingly complex. Consequently, the manual tracking of service health and availability proves to be a resource-intensive and error-prone endeavor. This is where the service registry becomes very useful.
The service registry is a database that keeps information on the services available. It provides a central point for service registration, lookup, and management.
When a microservice starts, it registers itself in the service registry. Other services that want to communicate use them as lookups or load balancing with lookups. It also updates the service instances record dynamically by removing and adding them.
3. @EnableDiscoveryClient Annotation
@EnableDiscoveryClient is a more generic annotation provided by Spring Boot, making it a flexible choice for service discovery.
We can think of it as a contract that allows our application to work with different service registries, both now and in the future. It comes bundled with the spring-cloud-commons dependency, making it a core part of Spring Cloud’s service discovery mechanism.
However, it doesn’t work on its own—we’ll need to add a specific dependency for the actual implementation, whether it’s Eureka, Consul, or Zookeeper.
4. @EnableEurekaClient Annotation
Eureka, originally developed by Netflix, provides a robust service discovery solution. Eureka Client is a fundamental component of Eureka.
Specifically, applications can function as clients, registering themselves with a service registry implemented using Eureka. Furthermore, Spring Cloud offers a valuable abstraction layer over it, and in conjunction with Spring Boot, it significantly simplifies the integration of Eureka into microservice architectures, thereby enhancing developer productivity.
Consequently, the @EnableEurekaClient annotation plays a pivotal role in this process. More precisely, it’s one of the annotations used for services acting as clients to register themselves to the Service registry.
4.1. Implementing Eureka Client
Eureka Client only works when we have the Eureka client dependency in the pom.xml file:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
We’d also need the spring-cloud-commons in the dependency management section in the same file:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>2021.0.5</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Once it’s registered, it provides metadata like host, URL, health URL, etc. Service registry also gets heartbeat messages from each service and if the heartbeat isn’t sent within a configurable timetable, the instance is removed from the service registry server.
We’ll now examine the essential Spring Boot properties required to configure a Eureka client, as they appear within the application.properties or application.yml file:
spring.application.name=eurekaClient
server.port=8081
eureka.client.service-url.defaultZone=http://localhost:8761/eureka/
eureka.instance.prefer-ip-address=true
Let’s understand the above properties:
- spring.application.name: This gives our application a name, a logical identifier (used for service discovery).
- server.port: Port number for this client at which it’s running.
- eureka.client.service-url.defaultZone: This is where the client finds the Eureka server. In essence, it’s the address of the service registry. So, ‘defaultZone’ is where the client knows how to register and fetch services.
- eureka.instance.prefer-ip-address: This property dictates that the Eureka client should register its IP address rather than its hostname.
4.2. Setting up Eureka Server
Before the Eureka Client gets registered, we also have to set up Eureka Server. For that, we need one more Spring Boot application having the @SpringBootApplication annotation, which is the foundation of any Spring Boot application, for setting up the basic configurations.
Consequently, @EnableEurekaServer annotation transforms our application into an Eureka server. In other words, it’s not just a client registering with a registry; it’s the registry. Therefore, this application becomes the central service discovery hub:
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Now, let’s include some properties in the application.properties file for configuring the Eureka Server:
spring.application.name=eurekaServer
server.port=8761
eureka.instance.hostname=localhost
eureka.client.register-with-eureka=false
eureka.client.fetch-registry=false
Let’s take a closer look at the above properties:
- spring.application.name: It gives our application a name tag
- server.port: Specifies the network port on which the Spring Boot application listens for incoming requests.
- eureka.instance.hostname: Defines the hostname that the Eureka server instance advertises during its registration process. In this configuration, localhost indicates that the server is executing on the local machine. In production environments, this property would be populated with the fully qualified domain name or IP address of the server.
- eureka.client.register-with-eureka: When set to false, it instructs the Eureka server instance to refrain from registering itself with another Eureka server
- eureka.client.fetch-registry: When set to false, it prevents the Eureka server instance from retrieving the service registry from another Eureka server
Once, the Eureka server is running, we can run the Eureka client too. And we’re ready with our implementation.
5. Choosing Between @EnableEurekaClient and @EnableDiscoveryClient
@EnableDiscoveryClient is the abstract interface that Spring Cloud Netflix puts above @EnableEurekaClient if future service registry tools are built. On the other hand, @EnableEurekaClient is for service discovery with Eureka.
Similarly, in other implementations like Eureka, Consul, Zookeeper, etc., we can have various combinations:
- @EnableDiscoveryClient with @EnableEurekaClient and service registry server as @EnableEurekaServer.
- @EnableDiscoveryClient with Consul and service registry server as Consul Server.
If our service registry is Eureka, both @EnableEurekaClient and @EnableDiscoveryClient can technically work for our clients. However, @EnableEurekaClient is generally recommended for its simplicity.
@EnableDiscoveryClient comes in handy in scenarios where we want more flexibility on the client side because it gives us more control.
It’s also worth noting that we’ll often see @EnableDiscoveryClient on a configuration class to enable discovery clients. But here’s a neat trick: if we’re using the Spring Cloud starter, we don’t need that annotation. Discovery client is enabled by default in that case. Plus – and this is cool if Spring Cloud finds the Netflix Eureka Client on our classpath – it automatically configures it for us.
Let’s sum up their main differences:
@EnableEurekaClient | @EnableDiscoveryClient |
---|---|
Enables service registration and discovery only with Eureka. |
More generic, works with multiple service registries |
It supports only Netflix Eureka. |
It supports Eureka, Consul, Zookeeper, etc. |
It’s a concrete implementation. |
It’s an interface or contract. |
It needs the
|
It’s a part of Spring Cloud dependencies. No specific dependency is needed. |
6. Conclusion
In this article, we saw how both @EnableEurekaClient and @EnableDiscoveryClient can work with Eureka. However, @EnableEurekaClient is generally the easier, more straightforward option if we know we’re using Eureka.
@EnableDiscoveryClient, on the other hand, is more versatile. It’s a good choice if we think we might use a different service registry in the future, or if we just want more flexibility and control. So, it all boils down to what we need and how much abstraction we want in our microservice setup.
As usual, the code for this tutorial is available over on GitHub.
The post EnableEurekaClient vs EnableDiscoveryClient: Which to Use? first appeared on Baeldung.