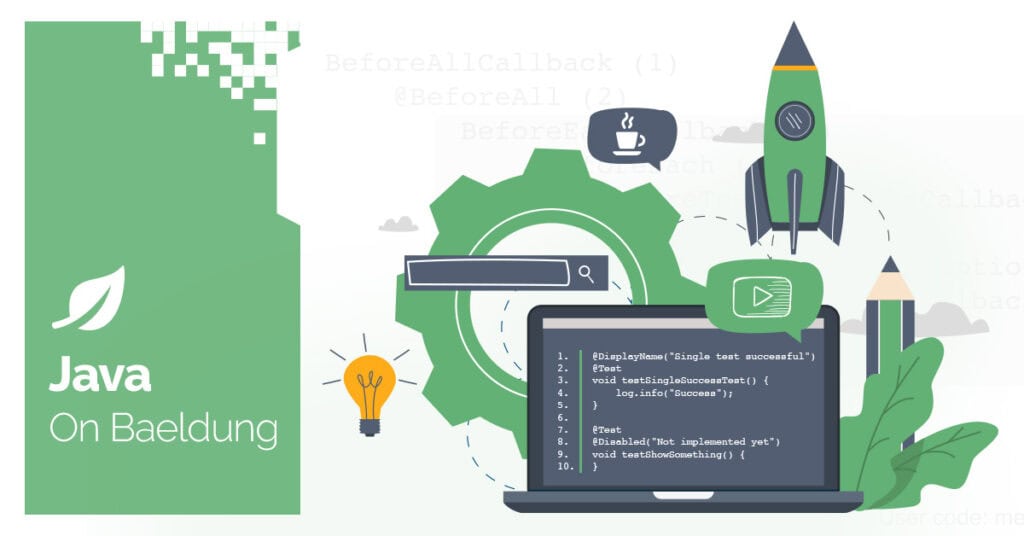
1. Overview
In this tutorial, we explore the essential Java naming conventions that help us write clear, maintainable, and consistent code. Adopting these conventions is crucial for reducing ambiguity and improving collaboration among developers.
We’ll delve into best practices for naming classes, interfaces, methods, variables, packages, enums, and annotations.
2. Class Naming Conventions
We name our classes using UpperCamelCase to indicate that the identifier represents a class. We choose names that are descriptive and use nouns or noun phrases to reflect the role or entity being modeled:
class CustomerAccount {
}
In this code, the class name CustomerAccount immediately tells us that the class is responsible for managing customer account information. We avoid abbreviations or vague names so that our code remains clear and self-explanatory.
3. Interface Naming Conventions
We name our interfaces using UpperCamelCase, just like classes. We choose names that clearly represent the behavior or contract expected from the implementing classes:
interface Printable {
}
In this example, the interface Printable defines a clear contract for printing functionality. We use a name that immediately indicates the purpose of the interface without any ambiguity.
4. Variable Naming Conventions
When naming variables, we focus on clarity and consistency to enhance code readability and maintainability. We choose descriptive names that reflect the variable’s purpose. For mutable fields, we use lowerCamelCase. On the other hand, for constants, we use UPPER_SNAKE_CASE:
public class CustomerAccount {
private String accountNumber;
private double balance;
public static final double MAX_BALANCE = 1000000.00;
}
In this example, accountNumber and balance are instance variables. The constant MAX_BALANCE is defined with the static final modifier and its name in UPPER_SNAKE_CASE.
5. Method Naming Conventions
We use lowerCamelCase for method names, ensuring they start with a verb that describes the action they perform. This practice makes our code intuitive and self-explanatory.
For instance, let’s consider the deposit() method in our CustomerAccount class:
public void deposit(double amount) {
if (balance + amount > MAX_BALANCE) {
System.out.println("Deposit exceeds max balance limit.");
} else {
this.balance += amount;
}
}
Here, the method name deposit() indicates that it adds an amount to the account balance.
Similarly, in our interface example, the print() method is named to reflect its behavior:
public interface Printable {
void print();
}
The method name print() concisely describes the action of producing output.
6. Package Naming Conventions
We organize our classes and interfaces into packages, which helps maintain structure. We follow a consistent naming pattern by using all lowercase letters and adopting reverse domain name notation:
package com.baeldung.namingconventions;
In this example, the package declaration follows the standard convention. This naming style not only organizes the code logically but also minimizes the risk of conflicts with other packages.
7. Enum Naming Conventions
We name the enum type using UpperCamelCase, while its constants are named in UPPER_SNAKE_CASE. This distinction helps us quickly identify that the enum represents a group of immutable values:
enum DayOfWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
}
In this example, the enum type DayOfWeek is written in UpperCamelCase. Each constant, such as SUNDAY or MONDAY, is in UPPER_SNAKE_CASE, which immediately communicates that these values are fixed and unchangeable.
8. Annotation Naming Conventions
We name annotation types using UpperCamelCase to indicate their role and purpose, just as we do with classes and interfaces:
@interface Auditable {
String action();
}
In this example, we define the annotation Auditable using UpperCamelCase, following the standard naming convention. This approach makes the code clear and easy to recognize, and when the annotation is applied, it provides specific metadata about the action related to a method or class.
9. Conclusion
In this article, we looked at the essential Java naming conventions for classes, interfaces, methods, variables, packages, enums, and annotations. We learned clear guidelines and examples that help us write code that is easy to read and maintain. By following these conventions, we create a consistent codebase that enhances collaboration and simplifies ongoing development.
As always, the source code is available over on GitHub.
The post Java Naming Conventions first appeared on Baeldung.