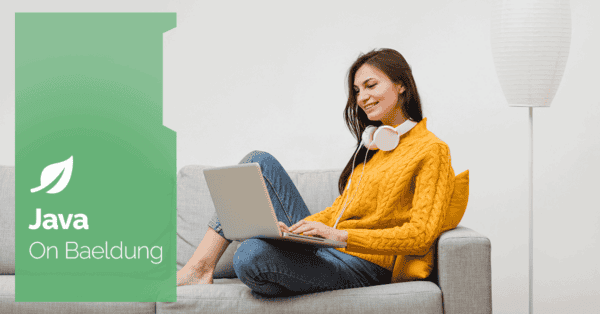
1. Introduction
As cloud technologies evolve, deploying and managing applications has become more seamless. However, this shift has also increased the need for robust operational data to monitor and maintain these applications effectively.
To achieve this, we require a control plane that provides visibility into an application’s health, performance, and runtime behavior. This is where the management interface comes into play – it offers a dedicated and secure endpoint for monitoring and managing applications efficiently.
In this tutorial, we’ll dive into the Management Interface in Quarkus, which enables developers and operations teams to access crucial runtime data such as health status, metrics, and application configurations.
2. Enabling and Configuring the Management Interface
By default, Quarkus doesn’t expose the management interface. However, once enabled, the management interface provides multiple endpoints for monitoring and diagnostics.
First, let’s enable it using the application.properties file of our Quarkus application:
quarkus.management.enabled=true
Then, we’ll restart our application using the Maven command:
mvn quarkus:dev
Now, our Quarkus application starts listening on port 9000 on all network interfaces (built-in configurations), keeping it separate from the main application endpoints:
2025-03-15 12:53:30,364 INFO [io.quarkus] (Quarkus Main Thread) quarkus-management-interface 1.0-SNAPSHOT
on JVM (powered by Quarkus 3.5.0) started in 1.173s.
Listening on: http://localhost:8080. Management interface listening on http://0.0.0.0:9000.
So, the default management interface endpoint is available at http://localhost:9000/q/ with /q as the default root path.
Also, Quarkus allows us to set the default settings like host, port, and root-path:
quarkus.management.host=localhost
quarkus.management.port=9090
quarkus.management.root-path=/management
Here, we’ve exposed the management interface on port 9090 of the local machine and the management interface endpoint will be http://localhost:9090/management.
For simplicity of this tutorial, we’ll use the default settings of the Quarkus management interface throughout.
3. Available Endpoints and Their Usage
Quarkus exposes several management endpoints that offer real-time insights into our application’s runtime state.
3.1. Application Info
First, let’s check our application’s info details by adding the latest quarkus-info Maven dependency to our pom.xml:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-info</artifactId>
<version>3.19.3</version>
</dependency>
Once added, we can retrieve our application details using the /q/info endpoint:
curl http://localhost:9000/q/info
The output would look like:
{
"java" : {
"version" : "21.0.6"
},
"os" : {
"name" : "Mac OS X",
"version" : "15.3.1",
"arch" : "aarch64"
},
"build" : {
"group" : "com.baeldung.quarkus",
"artifact" : "quarkus-management-interface",
"version" : "1.0-SNAPSHOT",
"time" : "2025-03-15T13:58:40.344217+05:30"
}
}
The JSON response provides information about the Java runtime, operating system, and build details of our Quarkus application.
3.2. Health Check
Now, let’s add the latest quarkus-smallrye-health Maven dependency to enable the health checks in our Quarkus application:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-smallrye-health</artifactId>
<version>3.19.3</version>
</dependency>
So, we can access application readiness:
curl http://localhost:9000/q/health/ready
A successful response would look like this:
{
"status": "UP",
"checks": []
}
Similarly, the application’s liveness would be available:
curl http://localhost:9000/q/health/live
Also, we can check the overall health status of our application:
curl http://localhost:9000/q/health
So, this endpoint combines checks for both readiness and liveness.
3.3. OpenAPI Documentation and Swagger UI
Quarkus provides endpoints for endpoints like /q/openapi and /q/swagger-ui to access OpenAPI and Swagger UI, respectively.
Let’s add the latest quarkus-smallrye-openapi Maven dependency:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-smallrye-openapi</artifactId>
<version>3.19.3</version>
</dependency>
Now, we can access the OpenAPI documentation of our application:
curl http://localhost:9000/q/openapi
This endpoint provides a comprehensive description of our application’s RESTful APIs in compliance with the OpenAPI specification.
Similarly, we can access the Swagger UI at http://localhost:9000/q/swagger-ui.
This provides an interactive user interface to explore and test our APIs.
3.4. Micrometer Metrics
Quarkus provides Micrometer metrics of our application along with Prometheus support.
First, let’s add the required quarkus-micrometer-registry-prometheus Maven dependency to our pom.xml:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-micrometer-registry-prometheus</artifactId>
</dependency>
Once added, we can access the Prometheus-compatible metrics:
curl http://localhost:9000/q/metrics
For instance, the response containing JVM and application metrics would look like:
# HELP worker_pool_completed_total Number of times resources from the pool have been acquired
# TYPE worker_pool_completed_total counter
worker_pool_completed_total{pool_name="vert.x-internal-blocking",pool_type="worker",} 0.0
worker_pool_completed_total{pool_name="vert.x-worker-thread",pool_type="worker",} 6.0
# HELP http_server_bytes_read Number of bytes received by the server
# TYPE http_server_bytes_read summary
http_server_bytes_read_count 0.0
http_server_bytes_read_sum 0.0
# HELP http_server_bytes_read_max Number of bytes received by the server
# TYPE http_server_bytes_read_max gauge
http_server_bytes_read_max 0.0
In essence, we retrieve a snapshot of our Quarkus application’s runtime health and performance.
Also, we can access Eclipse Microprofile-based metrics too using the quarkus-smallrye-metrics Maven dependency. However, it’s deprecated, and when Quarkus upgrades to Eclipse MicroProfile 6, SmallRye Metrics support will be discontinued.
4. Custom Endpoint on the Management Interface
Quarkus allows us to define a custom management endpoint on the management interface by registering routes on the management interface router.
For this, we’ll observe if the ManagementInterface event is fired and routed to our custom endpoint to return a response:
public void registerManagementRoutes(@Observes ManagementInterface mi) {
mi.router()
.get("/q/custom")
.handler(rc -> rc.response()
.end("Custom Management Endpoint Active"));
}
Here we registered a custom management endpoint /q/custom that responds with a String response:
curl http://localhost:9000/q/custom
The custom route will be triggered when the management interface is initialized to respond:
Custom Management Endpoint Active
This confirms that our custom endpoint is successfully registered and accessible within the Quarkus management interface.
5. Securing the Management Interface
Since the management interface exposes critical application details, we must have a way to secure it against unauthorized access.
To address this, Quarkus provides built-in authentication and authorization mechanisms for management interfaces too.
5.1. Basic Authentication
We can add the following configuration to enable basic authentication on our application’s management interface:
quarkus.management.auth.enabled=true
quarkus.management.auth.basic=true
When both properties are enabled, any request to a management endpoint requires authentication.
5.2. Role-Based Access Control
Quarkus also supports fine-grained access control using role-based authorization using the property quarkus.management.auth.roles-mapping.”role-name”:
quarkus.management.auth.roles-mapping.admin=true
quarkus.management.auth.roles-mapping.operator=true
This ensures that only users with admin or operator roles can interact with the management interface.
5.3. HTTPS Configuration
Enabling HTTPS ensures encrypted communication between clients and the management interface. So, we can also enable the recommended SSL for our management endpoints:
quarkus.management.ssl.certificate.key-store-file=keystore.jks
quarkus.management.ssl.certificate.key-store-password=secret
Hence, by configuring authentication, role-based access, and secure communication, we can effectively safeguard the Quarkus management interface from unauthorized access.
6. Conclusion
In this article, we explored the Management Interface in Quarkus and its role in monitoring and managing applications efficiently.
We discussed how to enable and configure the management interface, use built-in endpoints such as health checks, OpenAPI, and Micrometer metrics, and create a custom management endpoint.
Additionally, we looked at a few common ways to secure management endpoints, including authentication and role-based access control.
As always, the code for this article is available over on GitHub.
The post Management Interface in Quarkus first appeared on Baeldung.