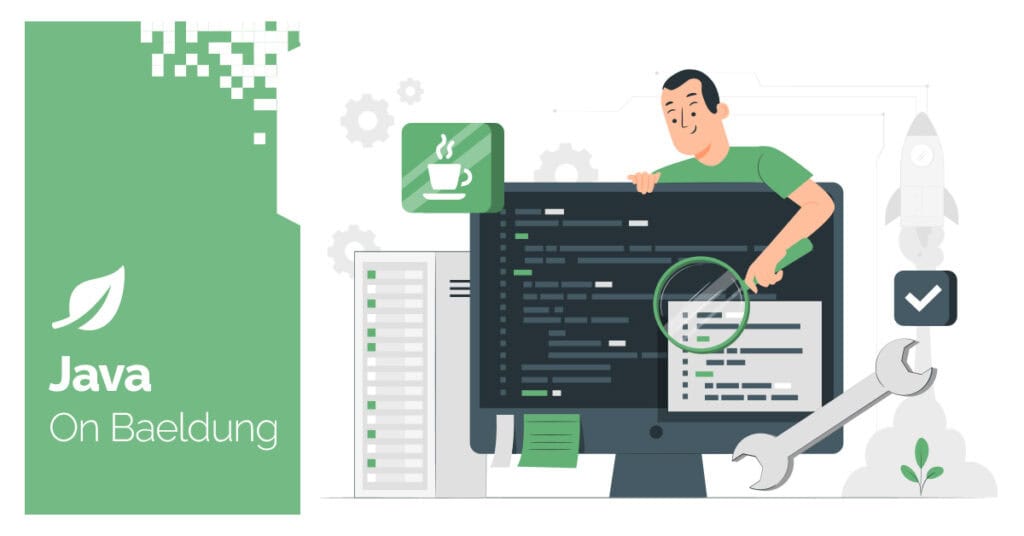
1. Introduction
In this tutorial, we’ll walk through the steps to integrate Google Translate API into a Java application. Google’s translation service supports over 100 languages, and by utilizing the API, we can easily build an application that performs real-time language translation.
2. Setting up the Java Application
To use the Google Translate API in our Java application, we need to include the necessary libraries.
We’ll be using the Google Cloud Translate client library, which provides an easy interface with which to interact with the API. This library will handle all the communication between our application and the Google Translate service.
To start, we need to add the following dependency to our pom.xml file. This includes the Google Translate client library in our project:
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-translate</artifactId>
</dependency>
This dependency ensures that we have access to the Google Cloud Translate API client library, which provides all the tools needed to send translation requests, detect languages, and manage API interactions efficiently.
3. Initializing the Translate Client
Once the dependency is added, the next step is to initialize the Translate client in our Java application. We’ll authenticate using a service account JSON file obtained from the Google Cloud Console. This approach is more secure and recommended for server-side applications.
Next, we should ensure that the service account has the Cloud Translation API enabled and assigned to the Cloud Translation API User role in Google Cloud.
The TranslateOptions class helps us set up the client with our API key, which then allows us to interact with the API service. Here’s how we can initialize the Translate client:
class Translator {
static {
initializeTranslateClient();
}
public static void initializeTranslateClient() {
if (translate == null) {
try {
GoogleCredentials credentials = GoogleCredentials.fromStream(
new FileInputStream("src/main/resources/YOUR_SERVICEACCOUNT_JSON.json")
);
translate = TranslateOptions.newBuilder()
.setCredentials(credentials)
.build()
.getService();
logger.info("Google Translate client initialized.");
} catch (Exception e) {
logger.error("Failed to initialize Google Translate client.", e);
}
}
}
// Additional methods will go here
}
In the code above, we use the TranslateOptions.newBuilder() method to configure the client with the service account credentials loaded using GoogleCredentials.fromStream(). After constructing the object, we call getService() to retrieve a Translate instance, which is then used to interact with the API.
4. Listing Supported Languages
The Google Cloud Translation API allows us to retrieve a list of supported languages. This is useful when displaying language options in a user interface or validating available languages before performing a translation.
We can fetch the list of supported languages using the listSupportedLanguages() method:
static void listSupportedLanguages() {
try {
List<Language> languages = translate.listSupportedLanguages();
for (Language language : languages) {
logger.info(String.format("Name: %s, Code: %s", language.getName(), language.getCode()));
}
} catch (Exception e) {
// handle exception
}
}
If we want the language names to be displayed in a specific target language, we can use the LanguageListOption.targetLanguage() method. For example, if we want the names to be displayed in Spanish (“es“):
List<Language> languages = translate.listSupportedLanguages(
Translate.LanguageListOption.targetLanguage("es")
);
for (Language language : languages) {
logger.info(String.format("Name: %s, Code: %s", language.getName(), language.getCode()));
}
This is useful when we want to present language options in a user’s preferred language rather than in English. When running the program, we shall see the output:
Name: Inglés, Code: en
Name: Español, Code: es
Name: Francés, Code: fr
Name: Chino (Simplificado), Code: zh
...
5. Translating Text
The Google Translate API offers a translate() method, which allows us to specify the text we want to translate and the target language. The API then returns the translated text.
In addition, the API also supports HTML input. When translating HTML, the API doesn’t modify any HTML tags, it only translates the text content between the tags.
Here’s how we can use the translate method to translate a piece of text:
static String translateText(String text, String targetLanguage) {
String s = "";
try {
Translation translation = translate.translate(
text,
Translate.TranslateOption.targetLanguage(targetLanguage)
);
s = translation.getTranslatedText();
} catch (Exception e) {
// handle exception
}
return s;
}
In this method, the text is the input that needs translation, and targetLanguage is the code of the language we want the text to be translated to (e.g., “es” for Spanish, “fr” for French). We then use translate.translate() to send the translation request, passing the text to be translated and the target language.
After receiving the translation response from the API, this method returns a Translation object, from which we can retrieve the translated text using getTranslatedText().
For example, if we call translateText(“Hello, world!”, “es”), the method returns “¡Hola, mundo!“. To ensure the translateText() method works as expected, we’ll write a unit test:
@Test
void whenTranslateTextIsCalledWithEnglishTextAndFrenchTargetLanguage_thenReturnTranslatedText() {
String originalText = "Hello, world!";
String targetLanguage = "es";
String expectedTranslatedText = "¡Hola Mundo!";
String translatedText = Translator.translateText(originalText, targetLanguage);
assertEquals(expectedTranslatedText, translatedText);
}
@Test
void whenTranslateTextIsCalledWithEnglishHTMLAndFrenchTargetLanguage_thenReturnTranslatedHTML() {
String originalHtml = "<p>Hello, world!</p>";
String targetLanguage = "es";
String expectedTranslatedHtml = "<p>¡Hola Mundo!</p>";
String translatedHtml = Translator.translateText(originalHtml, targetLanguage);
assertEquals(expectedTranslatedHtml, translatedHtml);
}
This test verifies that the translateText() method correctly translates the input text into the target language.
6. Detecting the Language of Text
The Google Translate API also supports language detection, which allows us to automatically identify the language of a given piece of text. This can be particularly useful if we don’t know the language of the input text.
The detection is straightforward and can be achieved with the detect() method, which returns the language code for the input text. Here’s how we can detect the language of a string:
static String detectLanguage(String text) {
return translate.detect(text).getLanguage();
}
In this example, we use translate.detect(text) to detect the language of the input text. The result is a Detection object, from which we extract the language using getLanguage().
For instance, if we call detectLanguage(“Hola, mundo!”), the method returns “es“, indicating that the text is in Spanish:
@Test
void whenDetectLanguageIsCalledWithSpanishText_thenReturnSpanishLanguageCode() {
String text = "Hola, mundo!";
String expectedLanguageCode = "es";
String detectedLanguage = Translator.detectLanguage(text);
assertEquals(expectedLanguageCode, detectedLanguage);
}
7. Translating Multiple Texts at Once
Additionally, If we need to translate multiple pieces of text, we can use the translate method with a list of strings. This approach is more efficient than making individual API calls for each text:
static List<String> translateBatch(List<String> texts, String targetLanguage) {
List<String> translationList = null;
try {
List<Translation> translations = translate.translate(
texts,
Translate.TranslateOption.targetLanguage(targetLanguage)
);
translationList = translations.stream()
.map(Translation::getTranslatedText)
.collect(Collectors.toList());
} catch (Exception e) {
// handle exception
}
return translationList;
}
This method takes a list of texts and a target language, translates all the texts in a single API call and returns a list of translated strings.
For example, if we call translateBatch(List.of(“Apple”, “Banana”, “Orange”), “fr”), the method returns List.of(“Pomme”, “Banane”, “Orange”):
@Test
void whenTranslateBatchIsCalledWithMultipleTexts_thenReturnTranslatedTexts() {
List<String> originalTexts = List.of("Apple", "Banana", "Orange");
List<String> expectedTranslatedTexts = List.of("Pomme", "Banane", "Orange");
List<String> translatedTexts = Translator.translateBatch(originalTexts, "fr");
assertEquals(expectedTranslatedTexts, translatedTexts);
}
8. Using a Custom Glossary
For specialized translations, the Google Cloud’s Translation v3 API supports glossaries, but we need to switch to TranslationServiceClient for that.
A glossary ensures that specific terms are translated consistently according to our preferences. For example, if we’re translating technical documentation, we might want to ensure that certain terms are always translated in a specific way.
To use a glossary, we first need to create one in the Google Cloud Console. Once we create it, we’ll receive a glossary ID, which we can use in our application:
static String translateWithGlossary(String projectId, String location, String text, String targetLanguage, String glossaryId) {
String translatedText = "";
try (TranslationServiceClient client = TranslationServiceClient.create()) {
LocationName parent = LocationName.of(projectId, location);
GlossaryName glossaryName = GlossaryName.of(projectId, location, glossaryId);
TranslateTextRequest request = TranslateTextRequest.newBuilder()
.setParent(parent.toString())
.setTargetLanguageCode(targetLanguage)
.addContents(text)
.setGlossaryConfig(TranslateTextGlossaryConfig.newBuilder()
.setGlossary(glossaryName.toString()).build()) // Attach glossary
.build();
TranslateTextResponse response = client.translateText(request);
translatedText = response.getTranslations(0).getTranslatedText();
} catch (IOException e) {
// handle exception
}
return translatedText;
}
In this method, we pass the glossary ID as an additional parameter to the translate() method. The API then uses the glossary to ensure that specific terms are translated according to our custom definitions.
For example, if we have a glossary that specifies “Hello” should be translated as “Salutations” in French, calling translateWithGlossary(“Hello”, “fr”, “glossary-id”) returns “Salutations“.
9. Conclusion
In this article, we’ve walked through the steps to integrate the Google Translate API into a Java application. We started by initializing the Translate client and implemented methods for translating text, detecting languages, translating multiple texts, and using custom glossaries.
As always, the source code is available over on GitHub.
The post Using Google Translate API in a Java Application first appeared on Baeldung.