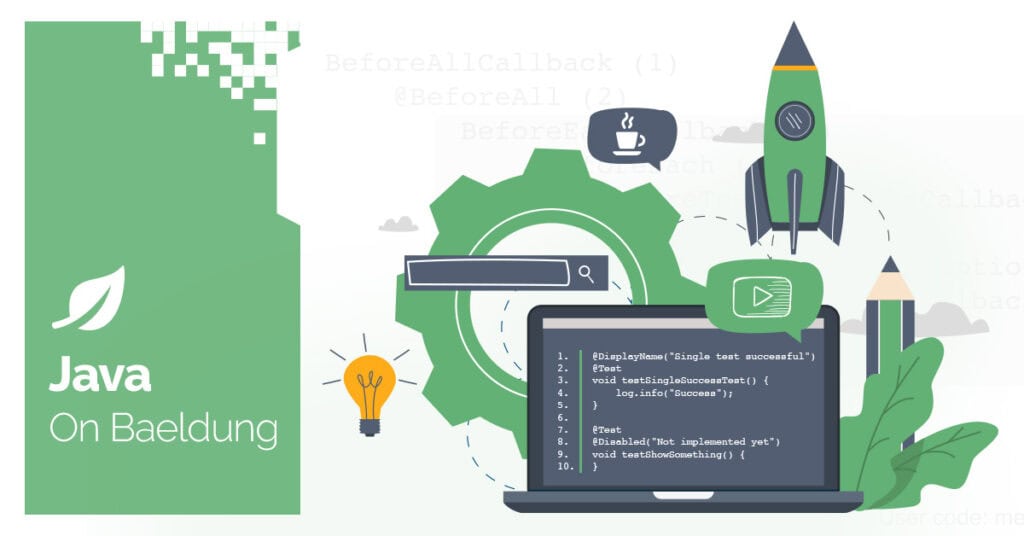
1. Introduction
In this tutorial, we’ll learn how to run or skip Scenarios selectively in Cucumber.
Cucumber is a tool that supports Behaviour-Driven Development (BDD). It reads executable specifications in plain text and validates that the software does what those specifications say.
2. Creating Scenarios
First, let’s create a few scenarios.
Let’s take an example of an API endpoint /greetings that generates a greeting message. We can write the following scenarios for it:
Feature: Time based Greeter
# Morning
Scenario: Should greet Good Morning in the morning
Given the current time is "0700" hours
When I ask the greeter to greet
Then I should receive "Good Morning!"
# Evening
Scenario: Should greet Good Evening in the evening
Given the current time is "1900" hours
When I ask the greeter to greet
Then I should receive "Good Evening!"
# Night
Scenario: Should greet Good Night in the night
Given the current time is "2300" hours
When I ask the greeter to greet
Then I should receive "Good Night!"
# Midnight
Scenario: Should greet Good Night at midnight
Given the current time is "0000" hours
When I ask the greeter to greet
Then I should receive "Good Night!"
Let’s now implement a controller method that generates a response as per the above specification:
@GetMapping("/greetings")
@ResponseBody
public String greet(@RequestParam("hours") String hours) {
String greeting;
int currentHour = Integer.parseInt(hours.substring(0, 2));
if (currentHour >= 6 && currentHour < 12) {
greeting = "Good Morning!";
} else if (currentHour >= 12 && currentHour < 16) {
greeting = "Good Afternoon!";
} else if (currentHour >= 16 && currentHour <= 19) {
greeting = "Good Evening!";
} else {
greeting = "Good Night!";
}
return greeting;
}
3. Approaches
Now, we’re ready to see how to ignore one or more of the scenarios we just defined.
3.1. Tagging With a Custom Tag
In this approach, we annotate our Scenarios with a custom annotation. It can be any string of our choice, e.g., @ignore, @skip or @disable. Let’s take something completely random say @custom-ignore to confirm that it doesn’t matter what we choose. However, something meaningful is a better choice.
Now, it’s a two-step process. First, we’ll mark the scenario with the annotation. Let’s mark the third scenario with this annotation:
# Night
@custom-ignore
Scenario: Should greet Good Night in the night
Given the current time is "2300" hours
When I ask the greeter to greet
Then I should receive "Good Night!"
Then, we’ll need to add the property cucumber.filter.tags in junit-platform.properties under the test resources folder:
cucumber.filter.tags=not @custom-ignore
And that’s it. This scenario will now be ignored in the subsequent test runs.
Notably, if we’re using Junit4, the same configuration needs to be specified using the @CucumberOptions annotation on a Cucumber TestRunner class:
@CucumberOptions(tags = "not @custom-ignore")
Additionally, we can also run only selective tags.
3.2. Commenting Out the Scenario
It’s worth noting that there are other options too, but they may not be as idiomatic as the first approach.
One such approach is to comment out the scenario:
# Night
# Scenario: Should greet Good Night in the night
# Given the current time is "2300" hours
# When I ask the greeter to greet
# Then I should receive "Good Night!"
However, this is error-prone as we might overlook commenting and uncommenting multiple lines.
4. Conclusion
In this article, we examined how using custom tags/annotations, we can reliably ignore one or more Scenarios in Cucumber.
Notably, Cucumber allows flexible tags to annotate and skip Scenarios. While we explored other options too, we learned that using a tag-based approach is the idiomatic way to achieve this goal.
As always, the complete source code of this tutorial is available over on GitHub.
The post How to Ignore Scenarios in Cucumber first appeared on Baeldung.