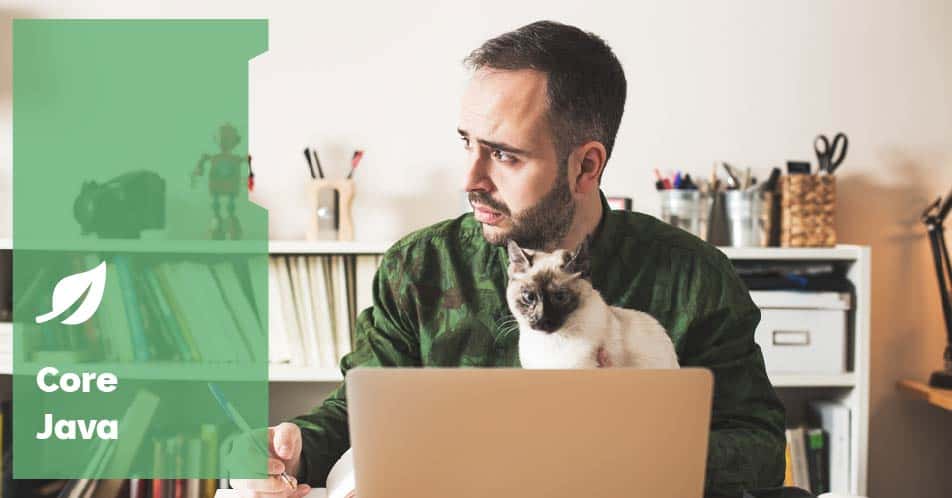
1. Overview
Unlike arrays, which allocate a contiguous block of memory for sequential storage, a linked list distributes its elements across non-contiguous memory locations, with each node referencing the next. The structure of a linked list allows for dynamic resizing, making it efficient for insertions.
In this tutorial, we’ll learn how to implement a custom singly linked list in Java with the functionality to insert, remove, retrieve, and count elements.
Notably, since the Java standard library provides a LinkedList implementation, our custom implementation is purely for educational purposes.
2. Understanding Linked List
A linked list is a collection of nodes, where each node stores a value and a reference to the next node in the sequence. The smallest unit of a linked list is a single node.
In a typical singly linked list, the first node is the head, and the last node is the tail. The tail’s reference to the next node is always null, marking the end of the list.
There are three common types of linked lists:
- Singly linked list – Each node points to the next node only
- Doubly linked list – Each node holds references to both the next and previous nodes
- Circular linked list – The last node’s reference points back to the head, forming a loop. It can be singly or doubly linked.
3. Creating a Node
Let’s create a class named Node:
class Node<T> {
T value;
Node<T> next;
public Node(T value) {
this.value = value;
this.next = null;
}
}
The class above represents a single element in a linked list. It has two fields:
- value – stores the data for this node
- next – holds a reference to the next node in the list
By using generics (<T>), we ensure that our linked list can store elements of any specified type.
4. Creating and Linking Nodes
Next, let’s see how nodes are linked together:
Node<Integer> node0 = new Node<>(1);
Node<Integer> node1 = new Node<>(2);
Node<Integer> node2 = new Node<>(3);
node0.next = node1;
node1.next = node2;
Here, we create three nodes of type Integer and link them together using the next reference. Then, let’s verify the structure with assertions:
assertEquals(1, node0.value);
assertEquals(2, node0.next.value);
assertEquals(3, node0.next.next.value);
These assertions confirm that each node correctly references the next in the sequence.
Notably, attempting to link nodes of different types results in a compilation error.
5. Implementing Linked List Operations
In the previous section, we implemented a basic Node class. Let’s build on that foundation by creating a custom singly linked list.
5.1. Defining the CustomLinkedList Class
First, let’s create a class named CustomLinkedList:
public class CustomLinkedList<T> {
private Node<T> head;
private Node<T> tail;
private int size;
public CustomLinkedList() {
head = null;
tail = null;
size = 0;
}
// ...
}
In the code above, the class holds references to the head and tail nodes.
When we create an empty object of CustomLinkedList, the head and tail are set to null. size helps keep track of the number of nodes. It’s set to zero by default.
5.2. Inserting Element at the Tail
Since a linked list can expand, let’s add a method to the CustomLinkedList to add a new node at the end of the list:
public void insertTail(T value) {
if (size == 0) {
head = tail = new Node<>(value);
} else {
tail.next = new Node<>(value);
tail = tail.next;
}
size++;
}
The method above accepts the value we intend to add as an argument. If the list is empty, both head and tail point to the new node.
Otherwise, the new node is linked to the existing tail, and tail is updated.
5.3. Inserting Element at the Head
Furthermore, let’s implement a method to insert a node at the beginning of the list:
public void insertHead(T value) {
Node<T> newNode = new Node<>(value);
newNode.next = head;
head = newNode;
if (size == 0) {
tail = newNode;
}
size++;
}
Here, the new node points to the current head, making it the new head. If the list is empty, tail is updated to point to the new node.
5.4. Retrieving an Element
Next, let’s define a method to retrieve a node’s values by its index:
public T get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of bounds");
}
Node<T> current = head;
for (int i = 0; i < index; i++) {
current = current.next;
}
return current.value;
}
In the code above, we validate the index to prevent out-of-bounds errors. Next, we traverse the list until we reach the specified index. Finally, we return the value stored at that node.
5.5. Removing the Head Node
To remove the first element, we simply update head to point to the next node:
public void removeHead() {
if (head == null) {
return;
}
head = head.next;
if (head == null) {
tail = null;
}
size--;
}
Here, we update head to point to the next node, effectively removing the old head. If the list becomes empty, tail is also set to null.
5.6. Removing a Node by Index
For more flexibility, let’s implement a method to remove a node at a specified index:
public void removeAtIndex(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of bounds");
}
if (index == 0) {
removeHead();
return;
}
Node<T> current = head;
for (int i = 0; i < index - 1; i++) {
current = current.next;
}
if (current.next == tail) {
tail = current;
}
current.next = current.next.next;
size--;
}
First, we remove the head using the removeHead() method if index is zero. Next, we traverse to the node before the target node. Then, we update the next pointer to skip the node being removed.
If we remove the last element, tail is updated.
5.7. Returning Size of the List
Finally, let’s add a method to return the size of the linked list:
public int size() {
return size;
}
Here, we return the size field that gets updated when we remove or add a node.
6. Conclusion
In this article, we learned how to create a custom linked list that mirrors Java’s built-in LinkedList. Also, we implemented insertion, retrieval, and removal methods for managing elements in our custom linked list.
As always, the complete source code for the examples is available over on GitHub.
The post Creating a Custom Linked List Data Structure in Java first appeared on Baeldung.