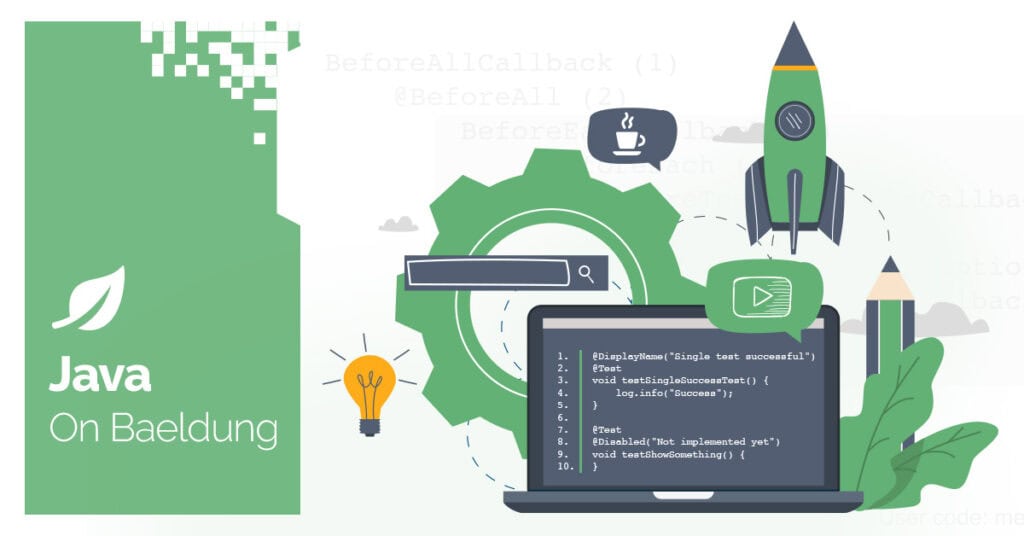
1. Overview
We often need to pass JVM options without modifying our scripts when configuring Java applications. Instead of manually adding flags every time we run the java command, we can use the environment variables JDK_JAVA_OPTIONS or JAVA_TOOL_OPTIONS. Both environment variables serve the same purpose—passing JVM options dynamically—but they work in different ways.
In this tutorial, we’ll explore their differences, when to use each, and best practices for managing JVM configurations effectively.
2. What Are JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS?
These two environment variables both allow us to specify JVM options globally, eliminating the need to modify the options every time we execute JDK tools such as java, javac, javadoc, etc.
JAVA_TOOL_OPTIONS was introduced in Java 5. On the other hand, JDK_JAVA_OPTIONS variable is more recent, introduced in Java 9. Their behavior and purpose are quite different.
Before we dive into how each variable functions, let’s create a simple Java source file:
package com.baeldung;
/**
* A simple class to print some variables' value
*/
public class TestEnvVar {
public static void main (String[] args){
System.out.println("var1 = '" + System.getProperty("var1") + "'");
System.out.println("var2 = '" + System.getProperty("var2") + "'");
}
}
The code above is pretty simple. Our main() method prints out the value of var1 and var2. Later, we’ll pass this argument to this class using these two environment variables.
Next, let’s compile the code:
$ javac com/baeldung/TestEnvVar.java
Now, we have the following file structure:
$ tree
.
└── com
└── baeldung
├── TestEnvVar.class
└── TestEnvVar.java
3 directories, 1 file
By the way, we’ll use Java 23 to compile or run all examples on Linux in this tutorial:
$ java -version
openjdk version "23.0.1" 2024-10-15
OpenJDK Runtime Environment Homebrew (build 23.0.1)
OpenJDK 64-Bit Server VM Homebrew (build 23.0.1, mixed mode, sharing)
Next, let’s run the class and pass some values to the required variables.
3. Executing the Java Launcher – the java Command
A typical way to start a Java program is using the Java launcher – the java command.
First, let’s define var1 and var2 in JDK_JAVA_OPTIONS and start our main() method:
$ JDK_JAVA_OPTIONS="-Dvar1='Hello (JDK_JAVA_OPTIONS)' -Dvar2='World (JDK_JAVA_OPTIONS)'" java com.baeldung.TestEnvVar
NOTE: Picked up JDK_JAVA_OPTIONS: -Dvar1='Hello (JDK_JAVA_OPTIONS)' -Dvar2='World (JDK_JAVA_OPTIONS)'
var1 = 'Hello (JDK_JAVA_OPTIONS)'
var2 = 'World (JDK_JAVA_OPTIONS)'
As the output indicates, the java command reads the JDK_JAVA_OPTIONS variable and passes the values of var1 and var2 to the main() method, producing the expected result. Also, java outputs a message to inform us that JDK_JAVA_OPTIONS was picked up.
It’s important to note that we set the environment variable and executed the java command on the same line, separated by a space. This ensures that JDK_JAVA_OPTIONS is only set for that specific java command. If we check the variable after running the command, we’ll see that JDK_JAVA_OPTIONS no longer holds a value:
$ echo $JDK_JAVA_OPTIONS
Now, let’s set var1 and var2 in JAVA_TOOL_OPTIONS and run our class:
$ JAVA_TOOL_OPTIONS="-Dvar1='Hi (JAVA_TOOL_OPTIONS)' -Dvar2='There (JAVA_TOOL_OPTIONS)'" java com.baeldung.TestEnvVar
Picked up JAVA_TOOL_OPTIONS: -Dvar1='Hi (JAVA_TOOL_OPTIONS)' -Dvar2='There (JAVA_TOOL_OPTIONS)'
var1 = 'Hi (JAVA_TOOL_OPTIONS)'
var2 = 'There (JAVA_TOOL_OPTIONS)'
Similarly, the JAVA_TOOL_OPTIONS variable affects the Java launcher. Also, a similar message tells us the java command read JAVA_TOOL_OPTIONS.
Now, some might wonder: what happens if the same variable is set in both JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS? Which one takes precedence? Next, let’s test it:
$ JAVA_TOOL_OPTIONS="-Dvar1='Hi (JAVA_TOOL_OPTIONS)' -Dvar2='There (JAVA_TOOL_OPTIONS)'" JDK_JAVA_OPTIONS="-Dvar2='World (by JDK_JAVA_OPTIONS)'" java com.baeldung.TestEnvVar
NOTE: Picked up JDK_JAVA_OPTIONS: -Dvar2='World (by JDK_JAVA_OPTIONS)'
Picked up JAVA_TOOL_OPTIONS: -Dvar1='Hi (JAVA_TOOL_OPTIONS)' -Dvar2='There (JAVA_TOOL_OPTIONS)'
var1 = 'Hi (JAVA_TOOL_OPTIONS)'
var2 = 'World (by JDK_JAVA_OPTIONS)'
In this example, we defined var1 and var2 in JAVA_TOOL_OPTIONS, but we only defined var2 with a different value in JDK_JAVA_OPTIONS. The output shows java picked up both environment variables. But if the same variables are set in both JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS, JDK_JAVA_OPTIONS takes precedence.
4. Other Java Commands
Apart from the java command, JDK provides several other commands that allow us to compile, document, and manage Java applications efficiently, such as:
- javac – the Java compiler
- javadoc – the JavaDoc generator
- and many more
Next, let’s figure out how these tools handle JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS.
We’ll take the javac compiler as an example. Unlike the java command, checking variable values through javac isn’t straightforward. Therefore, to test its behavior, we’ll set the Max Heap Size (-Xmx) JVM parameter in JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS, and verify whether it affects javac as expected.
First, let’s check the default MaxHeapSize value javac takes:
$ javac -J-XX:+PrintCommandLineFlags com/baeldung/TestEnvVar.java
... -XX:MaxHeapSize=9663676416 ...
In this example, the -J-XX:+PrintCommandLineFlags option is used with certain JDK tools, javac in this case, to print out the command-line flags that are being used by the JVM when the tool starts.
As the output shows, on this machine, JVM takes 9GiB (9*1024*1024*1024=9663676416 Bytes) memory as the default MaxHeapSize. Next, let’s set 10MiB (10*1024*1024 = 10485760 bytes) as MaxHeapSize in JAVA_TOOL_OPTIONS and compile the source file again:
$ JAVA_TOOL_OPTIONS="-Xmx10m" javac -J-XX:+PrintCommandLineFlags com/baeldung/TestEnvVar.java
Picked up JAVA_TOOL_OPTIONS: -Xmx10m
... -XX:MaxHeapSize=10485760 ...
As the output shows, JAVA_TOOL_OPTIONS affects javac.
Next, let’s do the same test with the JDK_JAVA_OPTIONS variable:
$ JDK_JAVA_OPTIONS="-Xmx10m" javac -J-XX:+PrintCommandLineFlags com/baeldung/TestEnvVar.java
... -XX:MaxHeapSize=9663676416 ...
It turns out that JDK_JAVA_OPTIONS doesn’t affect javac. It compiles the source file still using the default option: MaxHeapSize=9GiB.
Actually, this isn’t just javac. It applies to all JDK tools except the java command—only JAVA_TOOL_OPTIONS is recognized.
Next, let’s verify it using another JDK tool, the javadoc command:
$ JAVA_TOOL_OPTIONS="-Xmx10m" javadoc -J-XX:+PrintCommandLineFlags com/baeldung/TestEnvVar.java
Picked up JAVA_TOOL_OPTIONS: -Xmx10m
... -XX:MaxHeapSize=10485760 ...
$ JDK_JAVA_OPTIONS="-Xmx10m" javadoc -J-XX:+PrintCommandLineFlags com/baeldung/TestEnvVar.java | sed 's/ /\n/g' | grep MaxHeapSize
Loading source file com/baeldung/TestEnvVar.java...
... -XX:MaxHeapSize=9663676416 ...
As we can see, the command ignores the -Xmx10m parameter set in JDK_JAVA_OPTIONS, but correctly recognizes the value from the JAVA_TOOL_OPTIONS variable.
5. JDK_JAVA_OPTIONS vs. JAVA_TOOL_OPTIONS
So far, we discussed how these two environment variables work with JDK tools. Next, to have a clear overview, let’s summarize the differences in a table:
JAVA_TOOL_OPTIONS | JDK_JAVA_OPTIONS | |
---|---|---|
Purpose | Environment variable to pass JVM options and arguments to JDK tools | Environment variable to pass JVM options and arguments to the Java launcher to start a Java application |
Scope | It affects all JDK tools (java, javac, javadoc, jar, etc.) | It affects only the java command |
Works In | Java 5+ | Java 9+ |
Precedence for the Java launcher | The values in JDK_JAVA_OPTIONS overwrites the same options set in this variable. | Takes precedence. |
Since the JDK_JAVA_OPTIONS environment variable was introduced in Java 9 and is exclusive to the java command, it should be used when setting options for launching applications on Java 9 or later. However, if we need to set options globally across JDK tools, JAVA_TOOL_OPTIONS is the appropriate choice.
6. Conclusion
In this article, we’ve explored the differences between the JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS with practical examples.
We’ve also learned when to use each variable, helping us manage JVM options effectively across different JDK tools.
The post What Is the Difference Between JDK_JAVA_OPTIONS and JAVA_TOOL_OPTIONS? first appeared on Baeldung.