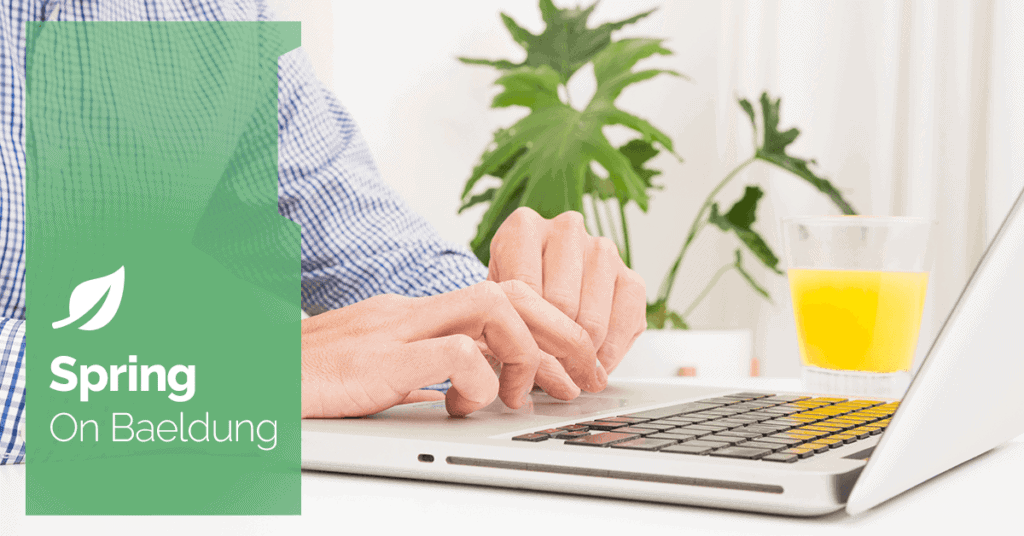
1. Overview
In Spring Boot projects that use Hibernate, entity validation is typically applied automatically during persistence.
While Hibernate’s built-in validation is useful, it can become redundant if our controllers already handle all necessary validation checks. This dual validation setup leads to duplicate validation, creating unnecessary overhead. Additionally, it can cause dependency injection issues when using custom validators that rely on Spring beans.
By disabling Hibernate validation specifically for JPA entities, we can prevent redundant checks and focus validation logic in the controller layer if our controllers already handle it effectively. This provides improved performance and a simplified codebase, as validation becomes centralized.
This tutorial covers the steps to disable Hibernate’s JPA entity validation, highlighting the benefits of this approach and its positive impact on application efficiency.
2. Why Disable Hibernate Validation?
Let’s explore the primary reasons for disabling Hibernate validation in Spring Boot applications. Although Hibernate’s automatic validation is convenient, it may be unnecessary if our controllers already handle validation effectively.
- Avoid Redundant Checks: When we handle validation fully in the controller, Hibernate’s entity-level validation duplicates this process, leading to wasted resources. Every time we persist an entity, Hibernate performs validation checks that the controller has already processed, resulting in unnecessary performance costs.
- Prevent Dependency Injection Issues: Hibernate validation can cause dependency injection issues, especially with custom validators. For instance, if a custom validator injects a Spring component (such as a repository to check uniqueness constraints), Hibernate validation will fail to recognize these injected dependencies. Disabling Hibernate validation enables these validators to operate smoothly within the Spring framework.
- Boost Performance: Disabling redundant Hibernate checks improves application performance. Relying solely on controller-based validation reduces the processing load during persistence, which is crucial for applications handling high transaction volumes or large data sets.
In short, if we already manage validation comprehensively within our controllers, disabling Hibernate’s validation makes our application leaner and more efficient.
3. Setting up Validation Exclusively in the Controller Layer
By configuring validation in the controller layer, we ensure that data is validated properly before it reaches the persistence layer. This approach keeps our validation centralized and provides better control over the flow of data into our application.
Let’s implement validation in our controller:
@PostMapping
public ResponseEntity<String> addAppUser(@Valid @RequestBody AppUser appUser, BindingResult result) {
if (result.hasErrors()) {
return new ResponseEntity<>(result.getFieldError().getDefaultMessage(), HttpStatus.BAD_REQUEST);
}
appUserRepository.save(appUser);
return new ResponseEntity<>("AppUser created successfully", HttpStatus.CREATED);
}
In this example, we use the @Valid annotation with a BindingResult parameter to capture validation errors directly in the controller. Let’s break this setup down:
- @Valid Annotation: triggers validation based on constraints defined on the AppUser entity (such as @NotNull, @Size, or custom annotations). This setup ensures that validation occurs at the controller level and not during persistence.
- BindingResult: This parameter captures any validation errors, allowing us to manage these errors before the entity is persisted. This way, if the AppUser entity fails validation, BindingResult will contain the error details, and we can handle them accordingly.
By setting up validation in the controller, we centralize all data checks at our application’s entry point, reducing the risk of invalid data reaching the database.
4. Disabling Hibernate Entity Validation in application.properties
To disable Hibernate validation for JPA entities, we modify the application.properties file in our Spring Boot project. By setting a single property, we disable Hibernate’s entity validation mechanism entirely, relying solely on our controller-based validations:
spring.jpa.properties.jakarta.persistence.validation.mode=none
This setting instructs Hibernate to skip entity validation during persistence. The jakarta.persistence.validation.mode property provides three options:
- auto: Validation runs automatically if Hibernate detects validation configurations.
- callback: Validation only occurs when explicitly triggered through a callback.
- none: This option disables Hibernate entity validation entirely, leaving validation to other application layers (in this case, our controller layer).
Setting this property to none instructs Hibernate to ignore any validation annotations on entities, allowing us to manage validation exclusively in the controller.
5. Custom Validators and Dependency Injection
When we disable Hibernate validation, we can confidently use custom validators that rely on Spring’s dependency injection. Custom validators provide a powerful way to enforce specific business rules or validation logic, particularly for unique constraints.
Let’s see an example of a custom validator:
public class UserUniqueValidator implements ConstraintValidator<UserUnique, String> {
@Autowired
private AppUserRepository appUserRepository;
@Override
public boolean isValid(String username, ConstraintValidatorContext context) {
return appUserRepository.findByUsername(username) == null;
}
}
This example shows a custom validator that checks for unique usernames by querying the repository. If Hibernate validation were active, this validator might fail due to dependency injection issues (for example, AppUserRepository might not be properly injected).
However, with Hibernate validation disabled, this validator operates independently within the controller, using Spring’s dependency injection to ensure that AppUserRepository is available.
6. Understanding the Difference Between Entity and Schema Validation
It’s essential to differentiate between entity validation (checking data constraints like @NotNull or @Size) and schema validation (synchronizing the entity structure with the database schema). Disabling Hibernate’s validation mode affects only entity-level validation, leaving schema validation unaffected.
Schema validation in Hibernate checks whether the database schema matches the entity mappings defined in the application. For example, if we change our entity classes (such as renaming a field or altering a column length), schema validation detects discrepancies between the entity definition and the database schema.
7. Disabling Schema Validation in application.properties
If we want Hibernate to skip schema validation as well (meaning it won’t validate or modify the database schema automatically), we adjust the corresponding property in our application.properties file:
spring.jpa.hibernate.ddl-auto=none
With this setting, Hibernate no longer executes schema validation or database creation/alteration operations. This setting is particularly useful if we manage the database schema independently with dedicated migration tools like Flyway or Liquibase.
8. Testing the Setup After Disabling Hibernate Validation
After configuring Hibernate to ignore entity validation, it’s essential to verify that validation functions correctly within the controller layer. This testing step ensures that our validations remain effective without Hibernate’s involvement.
Let’s review the steps we need to take for testing our setup:
- Functional Testing: Send various valid and invalid data inputs to the controller endpoints to confirm that validation errors are captured correctly. This step verifies that the controller-level validation setup is working as intended.
- Log Verification: Check the application logs to ensure that Hibernate validation errors are absent. All validation error messages should now originate from the controller, indicating that we’ve successfully disabled Hibernate validation.
This testing phase confirms that our application processes validation solely through the controller layer and that Hibernate does not reintroduce validation checks during persistence.
9. Managing Validation Exceptions Globally
Using @ControllerAdvice provides an effective way to handle validation errors globally in Spring Boot, ensuring consistent responses across the application.
Let’s look at an example of a global exception handler:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<String> handleValidationException(MethodArgumentNotValidException ex) {
return new ResponseEntity<>("Validation error: " + ex.getMessage(), HttpStatus.BAD_REQUEST);
}
}
With this setup, we can manage validation errors more effectively, delivering a clean and consistent error response for invalid data inputs. This approach enhances the user experience by clearly communicating validation errors.
10. Key Benefits of Disabling Entity Validation
By disabling entity validation, we gain several advantages that improve our application’s performance, maintainability, and overall efficiency:
- Centralized Validation Logic: With validation handled exclusively in the controller layer, all validation rules are managed in one place, simplifying the codebase and improving maintainability.
- Reduced Redundancy: Removing duplicate checks eliminates the risk of inconsistent validation results and prevents unnecessary processing.
- Enhanced Performance: Fewer validation checks lead to faster processing times, which is especially beneficial in high-traffic applications where performance is essential.
11. Conclusion
Disabling entity validation in Spring Boot is a practical optimization that streamlines validation management, boosts application performance, and minimizes complexity. By centralizing validation logic in the controller layer, we maintain robust data integrity while avoiding potential pitfalls related to dependency injection and redundant checks.
This approach offers a leaner, more efficient solution for applications with high-performance demands or complex validation rules, giving us better control over validation without compromising functionality.
The complete source code for this tutorial is available over on GitHub.