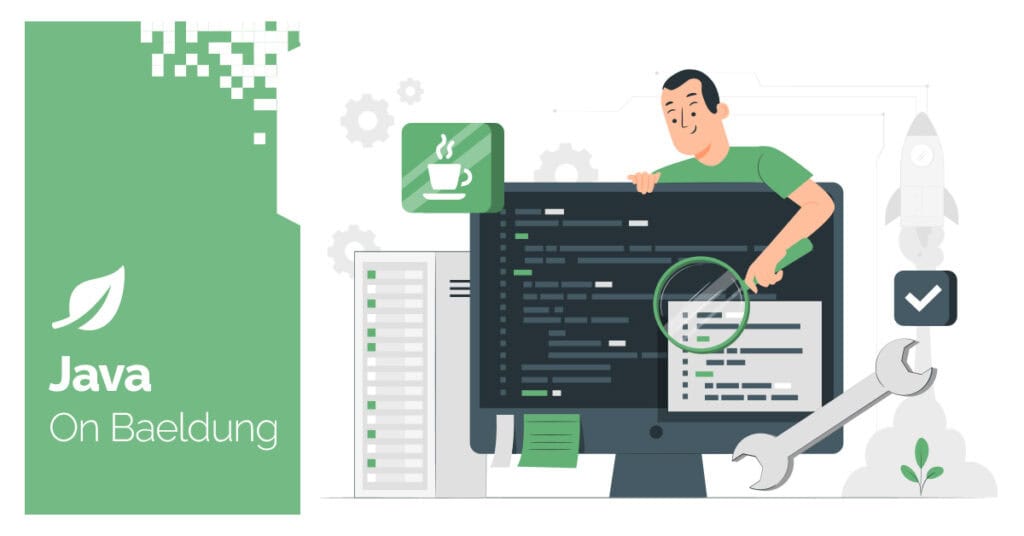
1. Overview
In Java programming, it’s common to face the need to convert a floating-point value to an integer. Since float allows decimal values, whereas int only holds whole numbers, converting between them can often lead to precision loss. It’s important to understand the different conversion techniques available to ensure our programs perform accurately.
In this article, we’ll look at various methods for converting a float to an int in Java, along with practical examples that demonstrate when each approach is appropriate.
2. float and int in Java
Let’s start by looking at the differences between float and int in Java:
- The float is a 32-bit data type used for representing numbers that have fractions. It follows the IEEE 754 standard and is suitable when we need a wide range of values, especially those involving decimals.
- On the other hand, int is also a 32-bit data type but only represents whole numbers. It’s perfect for tasks that require only integers, like counting items or representing positions in an array.
When converting a float to an int, we need to keep in mind that this conversion will remove the fractional part, leading to a potential loss of accuracy. The method we choose to convert a float to an int depends on how we want to handle that fractional portion.
3. Methods of float to int Conversion
There are several methods for converting a float to an int in Java. Each method handles the fractional component differently, and we need to pick the one that best suits our application’s requirements.
3.1. Explicit Type Casting
The most straightforward way to convert a float to an int is to use explicit type casting. By placing (int) in front of the float value, we forcefully convert it to an integer. This type of conversion simply removes the decimal part without rounding.
Let’s demonstrate this with a unit test:
@Test
public void givenFloatValues_whenExplicitCasting_thenValuesAreTruncated() {
int intValue1 = (int) 7.9f;
int intValue2 = (int) 5.4f;
int intValue3 = (int) -5.1f;
assertEquals(7, intValue1);
assertEquals(5, intValue2);
assertEquals(-5, intValue3);
}
In this example, we can see that the value 7.9 is converted to 7, the value 5.4 is converted to 5, and -5.1 is converted to -5. The decimal portion is truncated, which means this method is effective when we’re only interested in the integer part and don’t care about rounding.
3.2. Using Math.round()
If we need to convert a float to an int while rounding to the nearest whole number, we can use the Math.round() method. This method handles the decimal value appropriately and rounds up or down based on its value.
To demonstrate this with a unit test, we can use the following code:
@Test
public void givenFloatValues_whenRounding_thenValuesAreRoundedToNearestInteger() {
int roundedValue1 = Math.round(7.9f);
int roundedValue2 = Math.round(5.4f);
int roundedValue3 = Math.round(-5.1f);
// Then
assertEquals(8, roundedValue1);
assertEquals(5, roundedValue2);
assertEquals(-5, roundedValue3);
}
In this example, the value 7.9 is rounded to 8, the value 5.4 is rounded to 5, and -5.1 is rounded to -5. This approach is useful when we want the closest integer representation, allowing for a more accurate conversion without always rounding down or up.
3.3. Using Math.floor() and Math.ceil()
In scenarios where we need greater control over the rounding behavior, Java’s Math class provides two useful methods:
- The Math.floor() method consistently rounds a given float value down to the nearest whole number.
- Conversely, Math.ceil() rounds a float value up to the nearest whole number.
To illustrate how these methods can be used in practice, we can look at a couple of unit tests:
@Test
public void givenFloatValues_whenFlooring_thenValuesAreRoundedDownToNearestWholeNumber() {
int flooredValue1 = (int) Math.floor(7.9f);
int flooredValue2 = (int) Math.floor(5.4f);
int flooredValue3 = (int) Math.floor(-5.1f);
assertEquals(7, flooredValue1);
assertEquals(5, flooredValue2);
assertEquals(-6, flooredValue3);
}
@Test
public void givenFloatValues_whenCeiling_thenValuesAreRoundedUpToNearestWholeNumber() {
int ceiledValue1 = (int) Math.ceil(7.9f);
int ceiledValue2 = (int) Math.ceil(5.4f);
int ceiledValue3 = (int) Math.ceil(-5.1f);
assertEquals(8, ceiledValue1);
assertEquals(6, ceiledValue2);
assertEquals(-5, ceiledValue3);
}
In these tests:
- The Math.floor() method converts 7.9 to 7, 5.4 to 5, and -5.1 to -6.
- The Math.ceil() method converts 7.9 to 8, 5.4 to 6, and -5.1 to -5.
Utilizing these approaches allows us to maintain consistency in how our conversions are rounded. This control is important when the rounding direction affects functionality, such as defining thresholds or handling aspects of the user interface.
4. Potential Issues with Conversion
When converting a float to an int, there are a few issues that we should be mindful of:
4.1. Loss of Precision
A key concern is the loss of precision. Since float values can include decimal parts, converting them into int results in the fractional portion being discarded. This truncation can lead to inaccuracies, especially in applications where the exact value is important.
4.2. Overflow Concerns
Another possible issue is overflow. Though both float and int are 32-bit types, they represent different value ranges. A float can store very large numbers, while int values are limited to just over two billion. If we attempt to convert a float that exceeds this range into an int, we may encounter overflow, leading to incorrect or unexpected outcomes.
4.3. Rounding Decisions
Each conversion method has its rounding behavior, and understanding these differences is crucial. For instance, using (int) simply truncates the value, whereas methods like Math.round(), Math.floor(), and Math.ceil() follow different rounding rules. Choosing the correct method is important to prevent bugs or inaccuracies, especially when rounding requirements are strict.
5. Conclusion
Converting float values to int in Java is a routine operation, but it necessitates careful consideration to maintain precision.
Whether we opt for explicit casting to truncate the value, utilize Math.round() for rounding to the nearest integer, or employ Math.floor() and Math.ceil() to consistently round either down or up, each method serves a distinct purpose and carries its implications.
By choosing the right approach according to our specific requirements, we can ensure that our programs manage numeric data effectively and accurately.
As always, the code can be found over on GitHub.