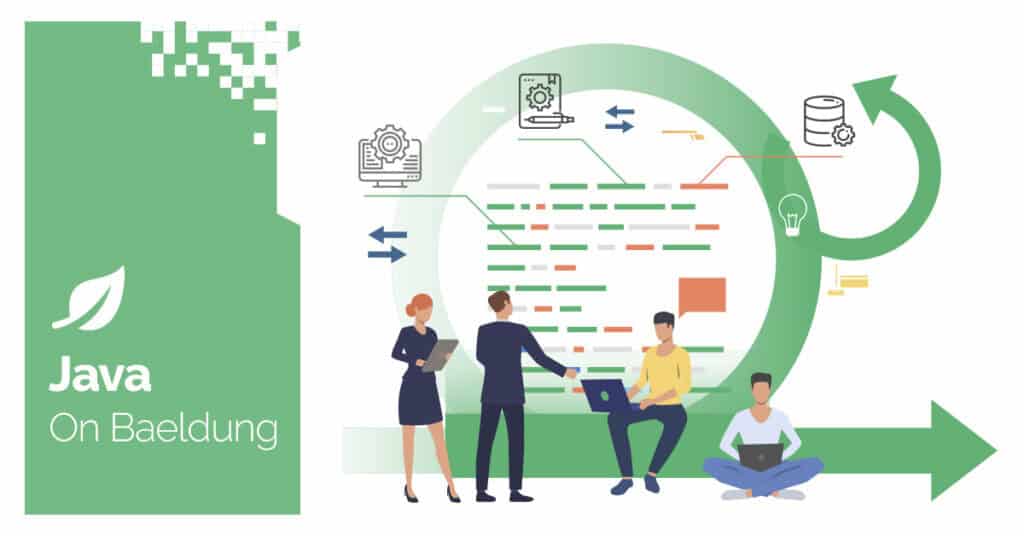
1. Introduction
In this article, we’ll explore how to read multiple integers from one line written in a file. We’ll explore the standard Java packages, specifically BufferedReader, and Scanner to achieve our desired result.
2. Sample Data
For all our examples, let’s define sample data and parameters. First, let’s start by manually creating a simple file named intinputs.txt in the resource folder, that we’ll use as an input source in our examples:
1 2 3 4 5
Furthermore, we’ll use the following sample values for the expected number of integers, and the file path:
private static final String FILE_PATH = "src/test/resources/intinputs.txt";
private static final int EXPECTED_NO_OF_INTEGERS = 5 ;
3. Using BufferedReader
Let’s explore the BufferedReader class which allows us to read input line by line. We can read the whole line into String and then based on the delimiter, we can split the input and parse them as Integers:
@Test
public void givenFile_whenUsingBufferedReader_thenExtractedIntegersCorrect() throws IOException {
try (BufferedReader br = Files.newBufferedReader(Paths.get(FILE_PATH))) {
String inputs = br.readLine();
int[] requiredInputs = Arrays.stream(inputs.split(" "))
.mapToInt(Integer::parseInt).toArray();
assertEquals(EXPECTED_NO_OF_INTEGERS, requiredInputs.length);
}
}
We created a stream on the split output using Arrays.stream() and then mapped them as Integer using the parseInt() method. As a result, we’ve read multiple Integers into an array from the input source.
4. Using Scanner
Using the Scanner class, we can achieve the same result with a similar approach. The Scanner class provides different methods to read data from the input source.
A token in a Scanner is defined by the delimiter pattern used by the Scanner to parse the input stream. By default, the delimiter pattern for a Scanner is any whitespace character (such as a space, tab, or newline).
We’ll specifically see nextInt() and nextLine() methods in detail.
4.1. Using the nextInt() Method
Scanner class provides the nextInt() method to read Integers from the input.
Let’s use the nextInt() method:
@Test
public void givenFile_whenUsingScannerNextInt_thenExtractedIntegersCorrect() throws IOException {
try (Scanner scanner = new Scanner(new File(FILE_PATH))) {
List<Integer> inputs = new ArrayList<>();
while (scanner.hasNext()){
inputs.add(scanner.nextInt());
}
assertEquals(EXPECTED_NO_OF_INTEGERS,inputs.size());
}
}
We’ve added the output of nextInt() method to the list of Integers. As a result, we’ve read multiple Integers from the input source. When working with nextInt(), we need to be sure that we only give it Integer inputs, otherwise, it throws InputMismatchException.
4.2. Using the nextLine() Method
Similar to BufferedReader, Scanner also provides a method to read the whole line. The nextLine() method reads the whole line and we can split it using the required delimiter:
@Test
public void givenFile_whenUsingScannerNextLine_thenExtractedIntegersCorrect() throws IOException {
try (Scanner scanner = new Scanner(new File(FILE_PATH))) {
String inputs = scanner.nextLine();
int[] requiredInputs = Arrays.stream(inputs.split(" "))
.mapToInt(Integer::parseInt).toArray();
assertEquals(EXPECTED_NO_OF_INTEGERS,requiredInputs.length);
}
}
Similar to BufferedReader, we created a stream on the split output using Arrays.stream() and then mapped them as Integer using the parseInt() method.
5. Conclusion
In this tutorial, we’ve explored different ways to read integers from the input using BufferedReader and Scanner methods. While BufferedReader has only one method to read input, the Scanner class provides specific methods to read primitive types.
As always, all of the code examples in this article are available over on GitHub.