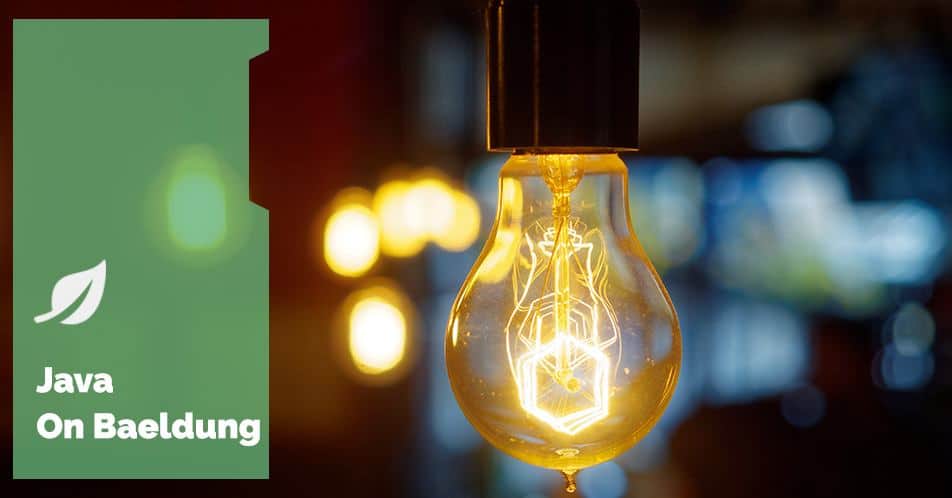
1. Overview
The Java JWT (JSON Web Token) library is a tool for creating, parsing, and validating JWT tokens. These tokens secure APIs and web applications and are a common solution for authentication and authorization.
In this tutorial, we’ll learn how to replace the deprecated parser().setSigningKey() method from this library with the more modern parserBuilder.setSigningKey(). Before diving into the implementation, we’ll discuss the reason for this change and the advantages of using JWT Parser-builder for parsing and validating JSON Web Tokens.
2. What Is the Signing Key?
The signing key is a crucial element of a JWT token. JWT tokens consist of three parts: a header, a payload, and a signature. The signature is created by signing the header and payload using a secret key or a public/private key pair. When a token is sent to the server, the signing key is used to validate the signature, ensuring that the token has not been tampered with.
In the Java JWT library, the signing key is provided to the parser during token validation. This ensures the token’s integrity and allows the server to trust its contents.
3. What Is the Jwts Class?
The Jwts class is a core utility class in the Java JWT library. It serves as the entry point for working with JWTs. This class provides us with various methods for creating, parsing, and validating JWTs. For instance, when we want to parse a JWT, we start by calling the Jwts.parserBuilder() method, which provides us with a builder for constructing a parser with the necessary configuration options, such as setting the signing key.
3.1 Deprecated Method Overview
Now that we have some context, let’s look at the deprecated parser().setSigningKey() method that we want to replace:
JwtParser parser = Jwts.parser().setSigningKey(key);
In this context, we use setSigningKey() to set the key for parsing and validating the JWT. This method was straightforward but had limitations, particularly regarding immutability and thread safety, which led to its eventual deprecation.
Specifically, this way of configuring the signing key was deprecated in version 0.11.0
4. Refactored Code With parserBuilder.setSigningKey()
To replace the deprecated method, we’ll use the JwtParserBuilder class instead. Furthermore, this was introduced in version 0.11.0 and provides us with a more secure and flexible API for handling JWT tokens. The new way to create our JwtParser with a signing key looks like this:
JwtParser parser = Jwts.parserBuilder()
.setSigningKey(key)
.build();
In this example, parserBuilder() allows us to build a parser with a signing key while offering additional configuration options. Finally, the build() method creates an immutable parser instance, ensuring that it’s thread-safe and allows reuse across different parts of our application.
5. Why the Change?
The deprecation of parser().setSigningKey() is part of a broader move toward enhancing security and flexibility in JWT handling. Let’s look at some of the key reasons for this change:
- Immutability and Thread Safety: The new parserBuilder.setSigningKey() method produces a JWT Parser instance that is immutable and thread-safe. This also means we can reuse the same parser across multiple threads without worrying about state capture and unexpected behavior.
- Enhanced Security: With parserBuilder.setSigningKey(), we can flexibly configure specific required claims and also set custom validation rules. This helps us enforce stricter security rules and easily customize our JWT validation process.
- Improved Readability: We can also improve the readability of our code by making configurations explicit and easier to understand with parserBuilder.setSigningKey().
6. Conclusion
In this article, we learned how to replace the deprecated parser().setSigningKey() method with the parserBuilder.setSigningKey(). We also explored the reasons behind the deprecation and discussed the benefits of using the updated API for creating JWT parsers.
As always, the code used in this tutorial is available over on GitHub.