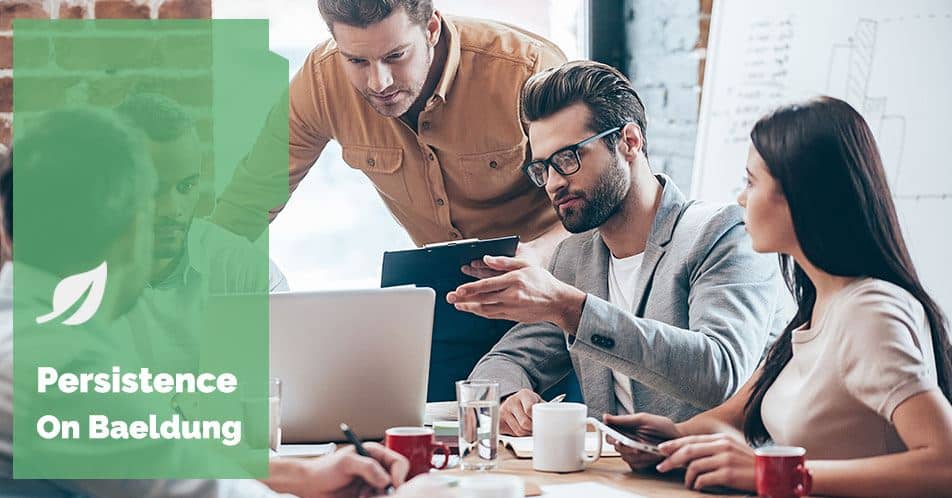
1. Introduction
Cloning a JPA entity means creating a copy of an existing entity. This allows us to make changes to a new entity without affecting the original object. In this tutorial, we’ll explore various approaches to clone a JPA entity.
2. Why Clone a JPA entity?
Sometimes, we want to copy data without modifying the original entity. For example, we may want to create a new record that is almost identical to an existing one, or we may need to safely edit an entity in memory without saving it to the database right away.
Cloning helps in these cases by allowing us to duplicate the entity and work on the copy.
3. Approaches to Clone
There are multiple strategies for cloning JPA entities, each affecting how thoroughly the original object and its associated entities are copied. Let’s explore each of them.
3.1. Using Manual Copying
The simplest approach to clone an entity is to manually copy its fields. We can either use a constructor or a method that explicitly sets each field value. This gives us full control over what is copied and how relationships are handled.
Let’s create the Product entity and Category entity classes:
@Entity
public class Category {
private Long id;
private String name;
// set and get
}
@Entity
public class Product {
private Long id;
private String name;
private double price;
private Category category;
// set and get
}
Here’s an example where we manually copy the fields of a Product entity using a method:
Product manualClone(Product original) {
Product clone = new Product();
clone.setName(original.getName());
clone.setCategory(original.getCategory());
clone.setPrice(original.getPrice());
return clone;
}
In the manualClone() method, we start by creating a new instance of the Product entity. Then, we explicitly copy each field from the original product to the new clone object.
However, when working with JPA, certain fields shouldn’t be copied during the cloning process. For instance, JPA typically auto-generates IDs, so copying the ID field could lead to issues when persisting the cloned entity.
Similarly, audit fields such as createdBy, createdDate, lastModifiedBy, and lastModifiedDate, which are used for tracking the creation and modification of entities, shouldn’t be copied. These fields should be reset to reflect the new clone’s lifecycle.
To verify the behaviour of our cloning method, we can write a simple test case:
@Test
void whenUsingManualClone_thenReturnsNewEntityWithReferenceToRelatedEntities() {
// ...
Product clone = service.manualClone(original);
assertNotSame(original, clone);
assertSame(original.getCategory(), clone.getCategory());
}
In this test, we see that the Category still refers to the same entity, indicating a shallow copy. If we want a deep copy of the Category, we need to clone it as well.
Here’s an example of how to deep clone the Category when cloning a Product:
Product manualDeepClone(Product original) {
Product clone = new Product();
clone.setName(original.getName());
// ... other fields
if (original.getCategory() != null) {
Category categoryClone = new Category();
categoryClone.setName(original.getCategory().getName());
// ... other fields
clone.setCategory(categoryClone);
}
return clone;
}
In this case, we create a new Category instance and manually copy its fields to achieve a deep clone.
Since we explicitly copy each field, we can determine exactly which fields should be duplicated and how they should be copied. However, it can become easy to overlook fields as the entity grows more complex.
3.2. Using Cloneable Interface
Another approach to cloning a JPA entity is by implementing the Cloneable interface and overriding the clone() method.
First, we need to ensure that our entity implements Cloneable:
@Entity
public class Product implements Cloneable {
// ... other fields
}
Then we override the clone() method in our Product entity:
@Override
public Product clone() throws CloneNotSupportedException {
Product clone = (Product) super.clone();
clone.setId(null);
return clone;
}
When we invoke the super.clone(), this method performs a shallow copy of the Product object.
3.3. Using Serialization
Another way to clone a JPA entity is to serialize it to a byte stream and then deserialize it back into a new object. This technique works well for deep cloning since it copies all fields and can even handle complex relationships.
To begin with, we need to ensure that our entity such as implements the Serializable interface:
@Entity
public class Product implements Serializable {
// ... other fields
}
Once our entity is serializable, we can proceed to clone it using the serialization method:
Product cloneUsingSerialization(Product original) throws IOException, ClassNotFoundException {
ByteArrayOutputStream byteOut = new ByteArrayOutputStream();
ObjectOutputStream out = new ObjectOutputStream(byteOut);
out.writeObject(original);
ByteArrayInputStream byteIn = new ByteArrayInputStream(byteOut.toByteArray());
ObjectInputStream in = new ObjectInputStream(byteIn);
Product clone = (Product) in.readObject();
in.close();
clone.setId(null);
return clone;
}
In this method, we first create a ByteArrayOutputStream to hold the serialized data. The writeObject() method converts the Product instance into a byte sequence and writes it to the ByteArrayOutputStream.
Next, an ObjectInputStream is then used to read the byte sequence and deserialize it back into a new Product object. Since JPA entities require unique identifiers, we explicitly set the ID to null to ensure that when we persist the cloned entity.
To verify this cloning method, we can write a test:
@Test
void whenUsingSerializationClone_thenReturnsNewEntityWithNewNestedEntities() {
// ...
Product clone = service.cloneUsingSerialization(original);
assertNotSame(original, clone);
assertNotSame(original.getCategory(), clone.getCategory());
}
In this test, the cloned Product is a distinct object from the original, including any nested entities like Category. It’s important to note that, nested entities like Category must also implement the Serializable interface.
Serialization is useful for deep cloning, as it copies all fields provided that all nested objects and relationships are also serializable.
However, serialization can be slower due to the overhead of converting objects to and from byte streams. Additionally, we must explicitly set the ID to null to avoid conflicts when persisting the cloned entity, as JPA expects a new ID for newly persisted entities.
3.4. Using BeanUtils
Additionally, we can also use the Spring Framework’s BeanUtils.copyProperties() method to clone an entity. This method copies the property values from one object to another. This approach is useful for shallow cloning where we need to quickly duplicate the properties of an entity without manually setting each one.
Before we can use this approach, we need to add the commons-beanutils dependency to our pom.xml:
<dependency>
<groupId>commons-beanutils</groupId>
<artifactId>commons-beanutils</artifactId>
<version>1.9.4</version>
</dependency>
Once the dependency is added, we can use BeanUtils.copyProperties() to implement the JPA entity cloning:
Product cloneUsingBeanUtils(Product original) throws InvocationTargetException, IllegalAccessException {
Product clone = new Product();
BeanUtils.copyProperties(original, clone);
clone.setId(null);
return clone;
}
BeanUtils is useful when we want a quick, shallow copy of an entity, though it doesn’t handle deep copying well. Therefore the category field won’t be copied.
Let’s verify this behaviour with a test case:
@Test
void whenUsingBeanUtilsClone_thenReturnsNewEntityWithNullNestedEntities() throws InvocationTargetException, IllegalAccessException {
// ...
Product clone = service.cloneUsingBeanUtils(original);
assertNotSame(original, clone);
assertNull(clone.getCategory());
}
In this example, it creates a new Product instance but doesn’t copy over the nested Category entity, leaving the Category as null.
Again, with this solution, we’re only performing a shallow copy. Fields such as ID and audit-related properties are intentionally excluded or reset, as this approach is designed to address quick entity duplication in a JPA-specific context.
3.5. Using ModelMapper
ModelMapper is another utility that can map one object to another. Unlike BeanUtils, which performs shallow copying, ModelMapper is designed to handle deep copying of complex, nested objects with minimal configuration. It’s highly effective when dealing with large entities that contain multiple fields or nested objects.
First, add the ModelMapper dependency to our pom.xml:
<dependency>
<groupId>org.modelmapper</groupId>
<artifactId>modelmapper</artifactId>
<version>3.2.1</version>
</dependency>
Here’s how we can use ModelMapper to clone a Product entity:
Product cloneUsingModelMapper(Product original) {
ModelMapper modelMapper = new ModelMapper();
modelMapper.getConfiguration().setDeepCopyEnabled(true);
Product clone = modelMapper.map(original, Product.class);
clone.setId(null);
return clone;
}
In this example, we use the modelMapper.map() method to clone the Product entity. This method maps the fields of the original Product object to a new Product instance.
Moreover, it recursively traverses through the object and its nested fields, performing a deep copy:
@Test
void whenUsingModelMapperClone_thenReturnsNewEntityWithNewNestedEntities() {
// ...
Product clone = service.cloneUsingModelMapper(original);
assertNotSame(original, clone);
assertNotSame(original.getCategory(), clone.getCategory());
}
In this test, we observe that the cloneUsingModelMapper() method creates a new Product instance and also a new Category instance for the cloned product.
3.6. Using JPA’s detach() Method
JPA provides a detach() method to detach an entity from the persistence context. After detaching the entity, we can modify it and save it as a new entity. This approach is useful when we want to make minimal changes to an entity and treat it as a new record in the database:
Product original = em.find(Product.class, 1L);
// Modify original product's name
original.setName("Smartphone");
em.merge(original);
original = em.find(Product.class, 1L);
em.detach(original);
original.setId(2L);
original.setName("Laptop");
Product clone = em.merge(original);
original = em.find(Product.class, 1L);
assertSame("Laptop", clone.getName());
assertSame("Smartphone", original.getName());
From the test case, we detach the original product instance from the persistence context. Once detached, any further modifications, such as updating the name, won’t be tracked automatically by JPA.
4. Conclusion
In this article, we’ve explored various approaches to cloning JPA entities. Manual copying is the most straightforward approach, providing full control over which fields to clone.
However, for more complex scenarios or when dealing with relationships, custom cloning methods or libraries like ModelMapper and BeanUtils can be beneficial.
As always, the code discussed here is available over on GitHub.