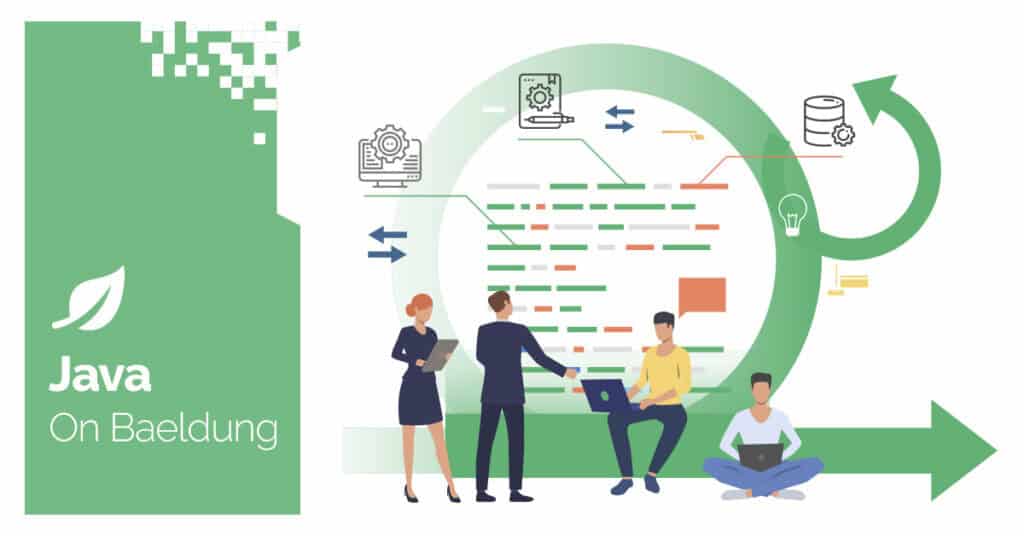
1. Overview
In Java, understanding how we call methods in static and non-static contexts is crucial, especially when working with methods like getClass().
One common problem we might encounter is trying to call the getClass() method from a static context, which will result in a compilation error.
In this tutorial, let’s explore why this happens and how we can handle it correctly.
2. Introduction to the Problem
The getClass() method is inherited from the Object class and returns the runtime Class of the object it is called upon. When we use getClass(), Java provides us with an instance of the Class object that represents the object’s runtime type.
Next, let’s see an example:
class Player {
private String name;
private int age;
public Player(String name, int age) {
this.name = name;
this.age = age;
}
public Class<?> currentClass() {
return getClass();
}
}
In this example, Player is a pretty straightforward class. The currentClass() method is an instance method, which allows us to obtain the Class<Player> object from a Player instance:
Player kai = new Player("Kai", 25);
assertSame(Player.class, kai.currentClass());
Sometimes, we want to get the current Class object from a static method. So, we may come up with this approach:
class Player {
// ... unrelated code omitted
public static Class<?> getClassInStatic() {
return getClass();
}
}
However, this code doesn’t compile:
java: non-static method getClass() cannot be referenced from a static context
Next, let’s understand why this happens and explore different ways to fix this problem.
3. Why Can’t We Call getClass() in a Static Context?
To figure out the cause of this problem, let’s quickly understand static and non-static contexts in Java.
A static method or variable belongs to the class instead of any particular class instance. This means that static members can be accessed without creating an instance of the class.
On the other hand, non-static members, such as instance methods and variables, are tied to individual instances of a class. We cannot access them without creating an object of the class.
Now, let’s examine why the compiler error occurred.
First, getClass() is an instance method:
public class Object {
// ... unrelated code omitted
public final native Class<?> getClass();
}
Our getClassInStatic() is a static method that we call without a Player instance. However, in this method, we invoked getClass(). The compiler raises an error because getClass() needs an instance of the class to determine the runtime type, but a static method doesn’t have any instance associated with it.
Next, let’s see how to resolve the issue.
4. Using the Class Literal
The most straightforward approach to retrieve the current Class object in a static context is to use the class literal (ClassName.class):
class Player {
// ... unrelated code omitted
public static Class<?> currentClassByClassLiteral() {
return Player.class;
}
}
As we can see, in the currentClassByClassLiteral() method, we return the Class object for the Player class using its class literal Player.class.
Since currentClassByClassLiteral() is a static method, it doesn’t rely on an instance. We can call it from the class:
assertSame(Player.class, Player.currentClassByClassLiteral());
As the test shows, using class literals is pretty straightforward for obtaining the class type in a static context. However, we must know the class name at compile time. It won’t help if we need to determine the class type dynamically at runtime.
Next, let’s see how to get the Class object in a static context dynamically.
5. Using MethodHandles
MethodHandle was introduced in Java 7 and is primarily used in the context of method handles and dynamic invocation.
The MethodHandles.Lookup class provides various reflection-like capabilities, and the lookupClass() method returns the Class object for which the lookup() method was performed.
Next, let’s create a static method in the Player class to return the Class object using this approach:
class Player {
// ... unrelated codes omitted
public static Class<?> currentClassByMethodHandles() {
return MethodHandles.lookup().lookupClass();
}
}
When we call this method, we get the expected Player.class object:
assertSame(Player.class, Player.currentClassByMethodHandles());
The lookupClass() method dynamically returns the calling class where the lookup() is performed. In other words, the lookupClass() method returns the class where the MethodHandles.Lookup instance was created. This can be useful when writing dynamic code that needs to inspect the current class reflectively at runtime.
Further, it’s worth noting that since it involves a dynamic lookup and reflection-like mechanisms, it is generally slower than the class literal approach.
6. Conclusion
Calling getClass() from a static context leads to a compilation error in Java. In this article, we’ve discussed the root cause of this error.
Additionally, we’ve explored two solutions to avoid this issue and obtain the expected Class object from a static context. Using class literals is straightforward and efficient. It’s the best choice if we have the class information at compile time. However, if we need to deal with a dynamic invocation at runtime, the MethodHandles approach is a helpful alternative.
As always, the complete source code for the examples is available over on GitHub.