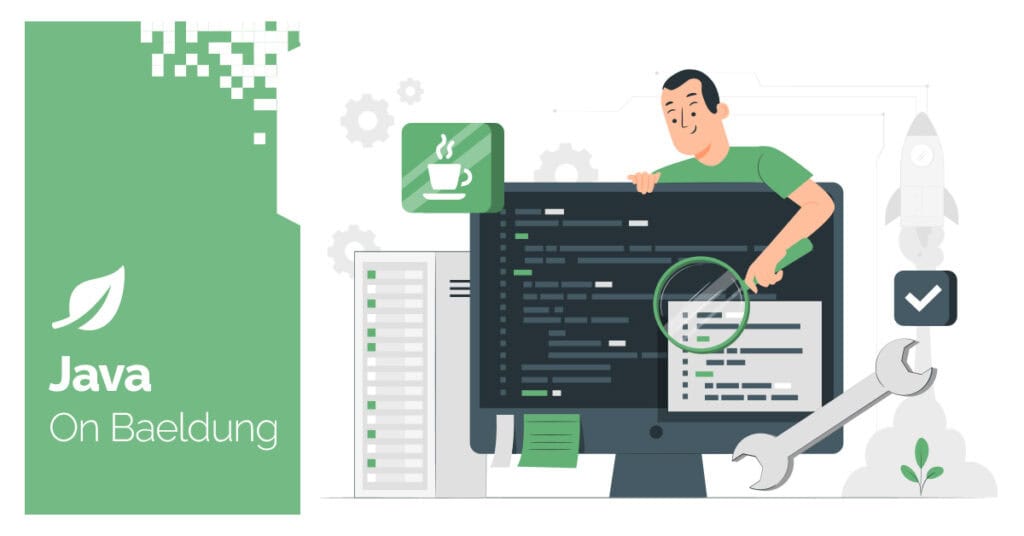
1. Overview
In this tutorial, we’ll explore two key methods in Java 8 Streams: findAny() and anyMatch(). Both methods serve different purposes, and understanding their differences is essential for writing effective Stream operations. After understanding these methods individually, we’ll compare findAny() and anyMatch() directly. This comparison will help clarify when to use each method based on the desired outcome.
We’ll briefly touch on related methods like findFirst(), count(), and allMatch(). These methods complement findAny() and anyMatch() in various scenarios, and we’ll get a quick overview of how they fit into the broader Stream API.
2. Understanding findAny()
We can use the findAny() method to retrieve any element from a Stream. It’s particularly useful when the order of elements doesn’t matter, as it’s designed to return an element without any guarantees about which one. This makes it a great choice for parallel streams, where performance can be prioritized over maintaining the order of elements.
The method returns an Optional, which means it can either hold a value or be empty if the Stream contains no elements. This is useful because it forces us to handle the possibility of an empty result, encouraging safer code practices.
Let’s take a look at a simple example to see findAny() in action:
@Test
public void whenFilterStreamUsingFindAny_thenOK() {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
Integer result = numbers.stream()
.filter(n -> n % 2 == 0)
.findAny()
.orElse(null);
assertNotNull(result);
assertTrue(Arrays.asList(2, 4, 6, 8, 10).contains(result));
}
In a sequential Stream like the one above, findAny() behaves similarly to findFirst(). It returns the first match it finds. However, the real advantage of findAny() comes when working with parallel streams, where it can quickly grab any matching element, potentially improving performance when order isn’t important:
@Test
public void whenParallelStreamUsingFindAny_thenOK() {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
Integer result = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.findAny()
.orElse(null);
assertNotNull(result);
assertTrue(Arrays.asList(2, 4, 6, 8, 10).contains(result));
}
By using a parallel Stream, findAny() can leverage parallelism to improve performance, especially for larger datasets.
3. Understanding anyMatch()
The anyMatch() method is used to check if any element in a Stream matches a given predicate. anyMatch() returns a boolean, true if any elements satisfy the condition, or false if none do. It’s ideal when we only need to check if any element meets a condition without retrieving the actual element.
anyMatch() is particularly efficient because it can short-circuit the Stream operation. As soon as it finds a matching element, it stops processing the rest of the Stream. If no elements match, the method evaluates the entire Stream. This behavior makes it useful when working with large datasets, as it doesn’t need to evaluate every element unnecessarily.
Let’s take a look at a simple example to see anyMatch() in action:
@Test
public void whenFilterStreamUsingAnyMatch_thenOK() {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
boolean result = numbers.stream()
.anyMatch(n -> n % 2 == 0);
assertTrue(result);
}
This method is great when we want a fast check to see if any element in a Stream satisfies a condition, without the need to process the entire Stream unless necessary.
4. Comparison Between findAny() and anyMatch()
While both findAny() and anyMatch() are useful when working with streams, they serve different purposes and return different types of results.
The next table provides a side-by-side comparison of both methods:
Feature | findAny() | anyMatch() |
---|---|---|
Return Type | Optional<T> | boolean |
Use Case | When we need to fetch an element without caring about the order | When we need to verify if at least one element matches |
Short-circuiting | Stops processing as soon as it finds a matching element | Stops processing as soon as it finds a match |
Parallel Stream | Efficient in parallel streams. Returns any element quickly | Works efficiently in parallel streams by checking conditions |
Null Safety | Returns an Optional to handle the absence of elements | Returns false if no matching elements are found |
5. Related Methods: findFirst(), count(), allMatch()
In addition to findAny() and anyMatch(), Stream offers other useful methods like findFirst(), count(), and allMatch() that serve different purposes when working with collections.
The findFirst() method returns the first element of the Stream, whether it’s processed sequentially or in parallel. It’s especially useful when element order matters, as it guarantees the first element in encounter order is returned:
@Test
public void whenFilterStreamUsingFindFirst_thenOK() {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
Integer result = numbers.stream()
.filter(n -> n % 2 == 0)
.findFirst()
.orElse(null);
assertNotNull(result);
assertEquals(2, result);
}
The count() method returns the total number of elements in a Stream:
@Test
public void whenCountingElementsInStream_thenOK() {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
long count = numbers.stream()
.filter(n -> n % 2 == 0)
.count();
assertEquals(5, count);
}
The allMatch() method checks if all elements in the Stream satisfy the provided predicate. If even one element fails the condition, it returns false. This method short-circuits as soon as it encounters an element that doesn’t match:
@Test
public void whenCheckingAllMatch_thenOK() {
List<Integer> numbers = Arrays.asList(2, 4, 6, 8, 10);
boolean allEven = numbers.stream()
.allMatch(n -> n % 2 == 0);
assertTrue(allEven);
}
Each of these methods has a specific purpose in Stream processing, allowing us to retrieve, count, or validate elements based on conditions.
6. Conclusion
In this article, we explored the differences between findAny() and anyMatch() and how they serve different purposes when working with streams.
While findAny() helps us retrieve an element from the Stream, anyMatch() is ideal for checking if any elements meet a condition. We also touched on related methods like findFirst(), count(), and allMatch(), which offer additional flexibility when processing Streams.
As always, the source code is available over on GitHub.