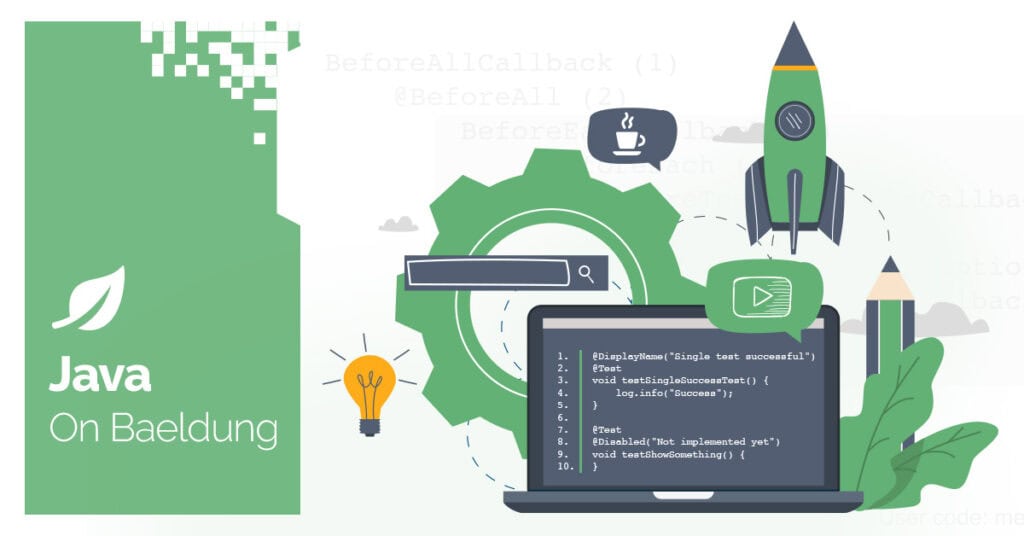
1. Introduction
In this tutorial, we’ll learn how to use Java JMS (Java Message Service) to read and write messages from IBM MQ queues.
2. Setting up the Environment
To avoid the complexities of manual installation and configuration, we can run IBM MQ inside a Docker container. We can use the following command to run the container with a basic configuration:
docker run -d --name my-mq -e LICENSE=accept -e MQ_QMGR_NAME=QM1 MQ_QUEUE_NAME=QUEUE1 -p 1414:1414 -p 9443:9443 ibmcom/mq
Next, we need to add the IBM MQ client in our pom.xml file:
<dependency>
<groupId>com.ibm.mq</groupId>
<artifactId>com.ibm.mq.allclient</artifactId>
<version>9.4.0.0</version>
</dependency>
3. Configuring JMS Connection
First, we need to set up a JMS connection with a QueueConnectionFactory, which is used to create connections to the queue manager:
public class JMSSetup {
public QueueConnectionFactory createConnectionFactory() throws JMSException {
MQQueueConnectionFactory factory = new MQQueueConnectionFactory();
factory.setHostName("localhost");
factory.setPort(1414);
factory.setQueueManager("QM1");
factory.setChannel("SYSTEM.DEF.SVRCONN");
return factory;
}
}
We start by creating an instance of MQQueueConnectionFactory, which is used to configure and create connections to the IBM MQ server. We set the hostname to localhost because the MQ server is running locally inside the Docker container. The port 1414 is mapped from the Docker container to the host machine.
Then we use the default channel SYSTEM.DEF.SVRCONN. This is a common channel for client connections to IBM MQ.
4. Writing Messages to IBM MQ Queue
In this section, we’ll go through the process of sending messages to an IBM MQ queue.
4.1. Establish a JMS Connection
To begin, we first create the MessageSender class. This class is responsible for setting up the connection to the IBM MQ server, managing the session, and handling message sending operations. We declare instance variables for QueueConnectionFactory, QueueConnection, QueueSession, and QueueSender, which will be used to interact with the IBM MQ server.
Below is an example implementation of the IBM MQ connection setup, session creation, and message sending:
public class MessageSender {
private QueueConnectionFactory factory;
private QueueConnection connection;
private QueueSession session;
private QueueSender sender;
public MessageSender() throws JMSException {
factory = new JMSSetup().createConnectionFactory();
connection = factory.createQueueConnection();
session = connection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = session.createQueue("QUEUE1");
sender = session.createSender(queue);
connection.start();
}
// ...
}
Next, in the constructor of the MessageSender, we initialize the QueueConnectionFactory using the JMSSetup class. This factory is then used to create a QueueConnection. This connection allows us to interact with the IBM MQ server.
Once the connection is established, we create a QueueSession using the createQueueSession(). This session allows us to communicate with the queue. Here we pass false to indicate the session is non-transactional and Session.AUTO_ACKNOWLEDGE to automatically acknowledge messages when they’re received.
After that, we define the specific queue “QUEUE1” and create a QueueSender to handle sending messages. Finally, we start the connection to ensure that the session is active and ready to transmit messages.
4.2. Writing a Text Message
Now that we have established a connection, created a session, defined the queue, and created a message producer, we’re ready to send a text message to the queue:
public void sendMessage(String messageText) {
try {
TextMessage message = session.createTextMessage();
message.setText(messageText);
sender.send(message);
} catch (JMSException e) {
// handle exception
} finally {
// close resources
}
}
First, we create a sendMessage() method that takes a messageText parameter. The sendMessage() method is responsible for sending a text message to the queue. It creates a TextMessage object and sets the message content using the setText() method.
Next, we send the message to the defined queue using the send() method of the QueueSender object. This design allows for efficient message transmission, as the connection and session remain open for as long as the MessageSender object exists.
4.3. Message Types
In addition to TextMessage, IBM MQ supports a variety of other message types that cater to different use cases. For instance, we can send the following:
- BytesMessage: A message holds raw binary data in the form of bytes.
- ObjectMessage: A message carries a serialized Java object.
- MapMessage: A message containing key-value pairs.
- StreamMessage: A message contains a stream of primitive data types.
5. Reading Messages From the IBM MQ Queue
Now that we’ve sent a message to the queue, let’s explore how to read messages from the queue.
5.1. Establish a JMS Connection and Create a Session
To begin, we need to establish a connection and create a session, similar to what we did when sending messages. We start by creating a MessageReceiver class. This class handles the connection to the IBM MQ server and sets up the components required for message consumption:
public class MessageReceiver {
private QueueConnectionFactory factory;
private QueueConnection connection;
private QueueSession session;
private QueueReceiver receiver;
public MessageReceiver() throws JMSException {
factory = new JMSSetup().createConnectionFactory();
connection = factory.createQueueConnection();
session = connection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = session.createQueue("QUEUE1");
receiver = session.createReceiver(queue);
connection.start();
}
// ...
}
In this class, we first create a QueueConnectionFactory to set up a connection to the IBM MQ server. We then use this connection to create a QueueSession, which allows us to interact with the queue.
Finally, we define the specific queue”QUEUE1” and create a QueueReceiver to handle incoming messages from the queue.
5.2. Reading a Text Message
Once the connection, session, and receiver are set up, we can start receiving messages from the queue. We use the receive() method of the QueueReceiver to pull messages from the specified queue:
public void receiveMessage() {
try {
Message message = receiver.receive(1000);
if (message instanceof TextMessage) {
TextMessage textMessage = (TextMessage) message;
} else {
// ...
}
} catch (JMSException e) {
// handle exception
} finally {
// close resources
}
}
In the receiveMessage() method, we use the receive() function to wait for a message from the queue, with a timeout of 1000 milliseconds. Once a message is received, we check if it’s of type TextMessage.
If it is, we can retrieve the actual message content using the getText() method, which returns the text content as a string.
6. Message Properties and Headers
In this section, we’ll discuss some commonly used message properties and headers that we can use when sending or receiving messages.
6.1. Message Properties
Message properties can be used to store and retrieve additional information beyond the message body. This is particularly useful for filtering messages or adding contextual data to the message. Here is how we can set the custom property when sending a message:
TextMessage message = session.createTextMessage();
message.setText(messageText);
message.setStringProperty("OrderID", "12345");
Next, we can retrieve the property when receiving a message:
Message message = receiver.receive(1000);
if (message instanceof TextMessage) {
TextMessage textMessage = (TextMessage) message;
String orderID = message.getStringProperty("OrderID");
}
6.2. Message Headers
Message headers provide predefined fields that include metadata about the message. Some commonly used message headers include:
- JMSMessageID: A unique identifier assigned by the JMS provider to each message. We can use this ID to track and log messages.
- JMSExpiration: Define the message expiration time (ms). If a message is not delivered within this time, it’ll be discarded.
- JMSTimestamp: The time the message was sent.
- JMSPriority: The priority of the message.
Let’s see how we can retrieve the message headers when receiving the message:
Message message = receiver.receive(1000);
if (message instanceof TextMessage) {
TextMessage textMessage = (TextMessage) message;
String messageId = message.getJMSMessageID();
long timestamp = message.getJMSTimestamp();
long expiration = message.getJMSExpiration();
int priority = message.getJMSPriority();
}
7. Mock Test With Mockito
In this section, we’ll use Mockito to mock dependencies and verify interactions for the MessageSender and MessageReceiver classes. We start by using the @Mock annotation to create mock instances of the dependencies.
Next, we verify the sendMessage() method correctly interacts with the mocked QueueSender. We mock the send() method of QueueSender, and verify the TextMessage is properly created:
String messageText = "Hello Baeldung! Nice to meet you!";
doNothing().when(sender).send(any(TextMessage.class));
messageSender.sendMessage(messageText);
verify(sender).send(any(TextMessage.class));
verify(textMessage).setText(messageText);
Lastly, we verify the receiveMessage() method correctly interacts with the mocked QueueReceiver. We mock the receive() method to return a pre-defined TextMessage and the message text is retrieved as we expected:
when(receiver.receive(anyLong())).thenReturn(textMessage);
when(textMessage.getText()).thenReturn("Hello Baeldung! Nice to meet you!");
messageReceiver.receiveMessage();
verify(textMessage).getText();
8. Conclusion
In this article, we explored the process of setting up JMS connections, sessions, and message producers/receivers for interacting with IBM MQ queues. We also introduced several message types supported by IBM MQ. Additionally, we highlighted how custom properties and headers can enhance message processing.
As always, the code discussed here is available over on GitHub.